java寻找数组最大值
时间: 2024-05-24 18:09:27 浏览: 94
要在Java中寻找数组的最大值,可以通过以下几种方式实现:
1. 使用循环遍历数组,逐一比较找到最大值。代码示例:
```
int[] arr = {1, 3, 5, 2, 4};
int max = arr;
for(int i = 1; i < arr.length; i++){
if(arr[i] > max){
max = arr[i];
}
}
System.out.println("最大值是:" + max);
```
2. 使用Java自带的Arrays工具类中的sort方法对数组进行排序,然后取最后一个元素即为最大值。代码示例:
```
int[] arr = {1, 3, 5, 2, 4};
Arrays.sort(arr);
int max = arr[arr.length - 1];
System.out.println("最大值是:" + max);
```
3. 使用Java8中新增的Stream API,通过max方法找到数组中的最大值。代码示例:
```
int[] arr = {1, 3, 5, 2, 4};
int max = Arrays.stream(arr).max().getAsInt();
System.out.println("最大值是:" + max);
```
相关问题
java输入数组寻找最大值
下面是一个示例代码,可以输入一个数组并找到其中的最大值:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入数组长度:");
int length = scanner.nextInt();
int[] arr = new int[length];
System.out.print("请输入数组元素:");
for (int i = 0; i < length; i++) {
arr[i] = scanner.nextInt();
}
int max = arr[0];
for (int i = 1; i < length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
System.out.println("数组中的最大值为:" + max);
}
}
```
以上代码首先输入数组的长度,然后输入数组的元素。接着使用一个循环遍历数组,找到其中的最大值并输出。
Java基于二分搜索如何寻找数组最大值
在Java中,二分查找通常用于已排序数组中查找特定元素,但并不直接适用于寻找最大值。然而,如果你有一个部分有序的数组,且想要利用这个特性来提高效率,这可能涉及到自定义逻辑。一般来说,寻找数组的最大值是通过遍历数组并不断更新当前找到的最大值来完成的,而不是通过二分查找。
下面是一个简单的Java程序,它不依赖于数组的排序性,而是通过循环遍历整个数组来找到最大值[^2]:
```java
import java.util.Scanner;
public class FindMax {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int[] num = new int[n];
int max = Integer.MIN_VALUE; // 初始化为整数最小值
for (int i = 0; i < num.length; i++) {
num[i] = sc.nextInt();
if (max < num[i]) { // 如果当前元素大于已知的最大值
max = num[i]; // 更新最大值
}
}
System.out.println("Max value: " + max);
}
}
```
阅读全文
相关推荐
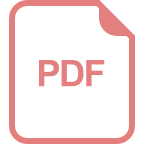
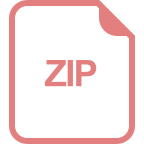
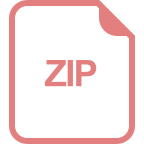













