请用python写一份火柴人格斗双人游戏
时间: 2023-07-18 15:43:04 浏览: 142
好的,下面是使用 Python 的 Pygame 库编写的一份火柴人格斗双人游戏的示例代码:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 设置窗口尺寸
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
# 设置窗口标题
pygame.display.set_caption("火柴人格斗")
# 加载图片
stickman_image = pygame.image.load("stickman.png")
# 设置火柴人的位置和速度
stickman1_pos = [100, 400]
stickman1_speed = [0, 0]
stickman2_pos = [600, 400]
stickman2_speed = [0, 0]
# 设置火柴人的大小和动作
stickman_size = [80, 120]
stickman1_rects = {"stand": pygame.Rect(0, 0, 20, 30),
"walk1": pygame.Rect(20, 0, 20, 30),
"walk2": pygame.Rect(40, 0, 20, 30),
"punch1": pygame.Rect(60, 0, 20, 30),
"punch2": pygame.Rect(80, 0, 30, 30)}
stickman2_rects = {"stand": pygame.Rect(0, 30, 20, 30),
"walk1": pygame.Rect(20, 30, 20, 30),
"walk2": pygame.Rect(40, 30, 20, 30),
"punch1": pygame.Rect(60, 30, 20, 30),
"punch2": pygame.Rect(80, 30, 30, 30)}
stickman1_action = "stand"
stickman2_action = "stand"
stickman1_flip = False
stickman2_flip = True
# 设置背景音乐和音效
pygame.mixer.music.load("bg_music.mp3")
pygame.mixer.music.play(-1)
punch_sound = pygame.mixer.Sound("punch.ogg")
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_a:
stickman1_speed[0] = -5
stickman1_flip = True
stickman1_action = "walk1"
elif event.key == pygame.K_d:
stickman1_speed[0] = 5
stickman1_flip = False
stickman1_action = "walk1"
elif event.key == pygame.K_j:
stickman1_action = "punch1"
punch_sound.play()
elif event.key == pygame.K_LEFT:
stickman2_speed[0] = -5
stickman2_flip = True
stickman2_action = "walk1"
elif event.key == pygame.K_RIGHT:
stickman2_speed[0] = 5
stickman2_flip = False
stickman2_action = "walk1"
elif event.key == pygame.K_KP1:
stickman2_action = "punch1"
punch_sound.play()
elif event.type == pygame.KEYUP:
if event.key == pygame.K_a or event.key == pygame.K_d:
stickman1_speed[0] = 0
stickman1_action = "stand"
elif event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
stickman2_speed[0] = 0
stickman2_action = "stand"
# 更新火柴人的位置
stickman1_pos[0] += stickman1_speed[0]
stickman1_pos[1] += stickman1_speed[1]
stickman2_pos[0] += stickman2_speed[0]
stickman2_pos[1] += stickman2_speed[1]
# 检测火柴人是否出界
if stickman1_pos[0] < 0:
stickman1_pos[0] = 0
elif stickman1_pos[0] > width - stickman_size[0]:
stickman1_pos[0] = width - stickman_size[0]
if stickman2_pos[0] < 0:
stickman2_pos[0] = 0
elif stickman2_pos[0] > width - stickman_size[0]:
stickman2_pos[0] = width - stickman_size[0]
# 绘制背景和火柴人
screen.fill((0, 0, 0))
screen.blit(stickman_image, stickman1_pos, stickman1_rects[stickman1_action])
screen.blit(pygame.transform.flip(stickman_image, stickman1_flip, False),
(stickman2_pos[0], stickman2_pos[1]), stickman2_rects[stickman2_action])
# 更新屏幕
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
在运行程序前需要准备好背景音乐、音效和火柴人的图片资源,并将代码中的文件名替换为你实际的文件名。运行程序后,你和你的朋友将会控制两个火柴人进行格斗,其中一个火柴人可以使用 A 键和 J 键进行移动和攻击,另一个火柴人可以使用左箭头和数字键 1 进行移动和攻击。如果想要结束程序,可以点击窗口右上角的关闭按钮。
相关推荐
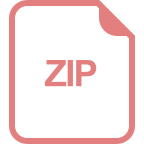














