用c语言写一个代码:参考课本迷宫问题的求解,用栈求解迷宫问题的所有路径以及最短路径。
时间: 2023-11-21 22:02:25 浏览: 55
以下是用栈求解迷宫问题的所有路径以及最短路径的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_ROW 10
#define MAX_COL 10
struct point {
int row, col;
} stack[512];
int top = 0;
void push(struct point p) {
stack[top++] = p;
}
struct point pop(void) {
return stack[--top];
}
int is_empty(void) {
return top == 0;
}
int maze[MAX_ROW][MAX_COL] = {
{0, 1, 0, 0, 0, 1, 0, 1, 0, 0},
{0, 1, 0, 1, 0, 1, 0, 1, 0, 1},
{0, 0, 0, 1, 1, 1, 1, 1, 0, 1},
{0, 1, 0, 0, 0, 1, 0, 0, 0, 0},
{0, 1, 1, 1, 0, 1, 1, 1, 1, 0},
{0, 1, 0, 1, 0, 0, 0, 0, 1, 0},
{0, 1, 0, 1, 1, 1, 1, 0, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 1, 1, 1, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
};
void print_maze(void) {
int i, j;
for (i = 0; i < MAX_ROW; i++) {
for (j = 0; j < MAX_COL; j++)
printf("%d ", maze[i][j]);
putchar('\n');
}
printf("*********\n");
}
void visit(int row, int col) {
struct point visit_point = { row, col };
maze[row][col] = 2;
push(visit_point);
}
int maze_path(int row, int col, int end_row, int end_col) {
struct point visit_point = { row, col };
maze[row][col] = 2;
push(visit_point);
if (row == end_row && col == end_col) {
printf("(%d, %d)\n", row, col);
return 1;
}
if (row > 0 && maze[row-1][col] == 0)
if (maze_path(row-1, col, end_row, end_col)) {
printf("(%d, %d)\n", row, col);
return 1;
}
if (row < MAX_ROW-1 && maze[row+1][col] == 0)
if (maze_path(row+1, col, end_row, end_col)) {
printf("(%d, %d)\n", row, col);
return 1;
}
if (col > 0 && maze[row][col-1] == 0)
if (maze_path(row, col-1, end_row, end_col)) {
printf("(%d, %d)\n", row, col);
return 1;
}
if (col < MAX_COL-1 && maze[row][col+1] == 0)
if (maze_path(row, col+1, end_row, end_col)) {
printf("(%d, %d)\n", row, col);
return 1;
}
pop();
return 0;
}
void maze_shortest_path(int row, int col, int end_row, int end_col) {
struct point visit_point = { row, col };
maze[row][col] = 2;
push(visit_point);
while (!is_empty()) {
struct point cur = pop();
if (cur.row == end_row && cur.col == end_col) {
struct point p;
printf("(%d, %d)\n", cur.row, cur.col);
while (!is_empty()) {
p = pop();
printf("(%d, %d)\n", p.row, p.col);
}
return;
}
if (cur.row > 0 && maze[cur.row-1][cur.col] == 0) {
visit(cur.row-1, cur.col);
}
if (cur.row < MAX_ROW-1 && maze[cur.row+1][cur.col] == 0) {
visit(cur.row+1, cur.col);
}
if (cur.col > 0 && maze[cur.row][cur.col-1] == 0) {
visit(cur.row, cur.col-1);
}
if (cur.col < MAX_COL-1 && maze[cur.row][cur.col+1] == 0) {
visit(cur.row, cur.col+1);
}
}
printf("No path found.\n");
}
int main(void) {
print_maze();
printf("The path is:\n");
maze_path(0, 0, 9, 9);
printf("*********\n");
printf("The shortest path is:\n");
maze_shortest_path(0, 0, 9, 9);
return 0;
}
```
该代码中,栈被用来记录路径上的点。maze数组表示迷宫,其中0表示可以通过的点,1表示障碍物,2表示已经访问过的点。迷宫的起点为(0, 0),终点为(9, 9)。maze_path函数用递归实现了深度优先搜索,寻找一条从起点到终点的路径,并打印出该路径。maze_shortest_path函数用广度优先搜索寻找起点到终点的最短路径,并打印出该路径。
阅读全文
相关推荐
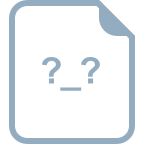

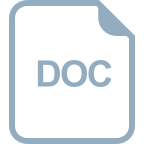


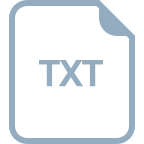
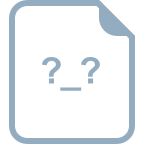
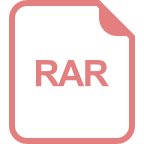
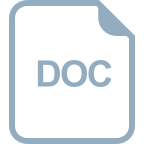
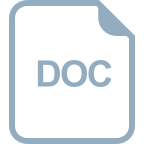
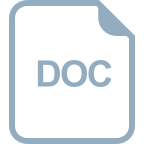
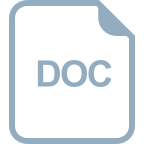
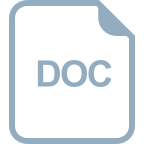
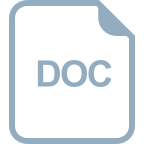
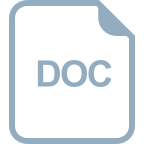
