@RequestMapping("/systemChange") public String systemChange(String id) { Student student = studentService.getById(id); LambdaQueryWrapper<Student> studentLambdaQueryWrapper = new LambdaQueryWrapper<>(); studentLambdaQueryWrapper.eq(Student::getSex,student.getSex()); //存在班级就寻找班级的学生 if (!ObjectUtils.isEmpty(student.getGrade())){ studentLambdaQueryWrapper.eq(Student::getCollege,student.getGrade()); } //并且存在宿舍的 studentLambdaQueryWrapper.isNotNull(Student::getDormitoryId); List<Student> studentList = studentService.list(studentLambdaQueryWrapper); //查出自己的文件答案 LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper = new LambdaQueryWrapper<>(); topicResultLambdaQueryWrapper.eq(TopicResult::getStuNum, student.getStudentNumber()) .and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2)); List<TopicResult> topicResults = topicResultService.list(topicResultLambdaQueryWrapper); for (Student student1 : studentList) { LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper1 = new LambdaQueryWrapper<>(); topicResultLambdaQueryWrapper1.eq(TopicResult::getStuNum, student1.getStudentNumber()) .and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2)); List<TopicResult> results = topicResultService.list(topicResultLambdaQueryWrapper1); if (topicResults.get(0).getOptionId().equals(results.get(0).getOptionId()) || topicResults.get(1).getOptionId().equals(results.get(1).getOptionId()) ){ Dormitory dormitory = dormitoryService.getById(student1.getDormitoryId()); if (dormitory.getNum()<dormitory.getTotal()){ student.setDormitoryId(dormitory.getId()); studentService.updateById(student); dormitory.setNum(dormitory.getNum()+1); dormitoryService.updateById(dormitory); return "redirect:list"; } } }请详细解释每一行代码
时间: 2023-06-25 19:05:46 浏览: 58
这是一个 Spring Boot 项目中的一个 Controller 类中的一个方法,访问路径为 "/systemChange"。
```
@RequestMapping("/systemChange")
public String systemChange(String id) {
```
这个方法接受一个 String 类型的参数 id,用来查找一个学生对象。
```
Student student = studentService.getById(id);
```
根据 id 查找到对应的学生对象。
```
LambdaQueryWrapper<Student> studentLambdaQueryWrapper = new LambdaQueryWrapper<>();
studentLambdaQueryWrapper.eq(Student::getSex,student.getSex());
```
使用 Mybatis-Plus 提供的 LambdaQueryWrapper 对象构建查询条件,这里查询条件为:性别与当前学生对象相同。
```
if (!ObjectUtils.isEmpty(student.getGrade())){
studentLambdaQueryWrapper.eq(Student::getCollege,student.getGrade());
}
```
如果当前学生对象的班级不为空,则将查询条件加上班级等于当前学生对象班级的限制。
```
studentLambdaQueryWrapper.isNotNull(Student::getDormitoryId);
```
加上查询条件:宿舍 ID 不为空,即只查找已分配宿舍的学生。
```
List<Student> studentList = studentService.list(studentLambdaQueryWrapper);
```
使用 Mybatis-Plus 提供的 Service 对象(studentService)进行查询,返回符合条件的学生列表。
```
LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper = new LambdaQueryWrapper<>();
topicResultLambdaQueryWrapper.eq(TopicResult::getStuNum, student.getStudentNumber())
.and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2));
List<TopicResult> topicResults = topicResultService.list(topicResultLambdaQueryWrapper);
```
使用 Mybatis-Plus 提供的 LambdaQueryWrapper 对象构建查询条件,这里查询条件为:学号等于当前学生对象的学号,并且题目 ID 为 1 或 2。查询结果为当前学生对象的文件答案。
```
for (Student student1 : studentList) {
LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper1 = new LambdaQueryWrapper<>();
topicResultLambdaQueryWrapper1.eq(TopicResult::getStuNum, student1.getStudentNumber())
.and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2));
List<TopicResult> results = topicResultService.list(topicResultLambdaQueryWrapper1);
if (topicResults.get(0).getOptionId().equals(results.get(0).getOptionId())
|| topicResults.get(1).getOptionId().equals(results.get(1).getOptionId())
){
Dormitory dormitory = dormitoryService.getById(student1.getDormitoryId());
if (dormitory.getNum()<dormitory.getTotal()){
student.setDormitoryId(dormitory.getId());
studentService.updateById(student);
dormitory.setNum(dormitory.getNum()+1);
dormitoryService.updateById(dormitory);
return "redirect:list";
}
}
}
```
遍历符合条件的学生列表,对于每个学生,使用与上面相同的查询条件,查询出该学生的文件答案。如果当前学生对象的文件答案与该学生的文件答案有一个相同,则判断该学生所在的宿舍是否还有空余床位,如果有,则将当前学生对象的宿舍 ID 设为该宿舍 ID,更新到数据库中,并将该宿舍的已分配床位数量加一,更新到数据库中。最后返回重定向到列表页面的字符串。
相关推荐
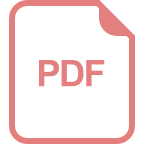
















