首先编写一个函数 void printInt(int n, int base), 功能是输出 n 的 base 进制表示
时间: 2023-12-21 11:03:49 浏览: 218
好的,我已经记录下来了。下面是函数的实现代码:
```c++
#include <iostream>
using namespace std;
void printInt(int n, int base) {
if (n < 0) {
cout << "-";
n = -n;
}
if (n >= base) {
printInt(n / base, base);
}
int digit = n % base;
if (digit >= 10) {
cout << (char)('A' + digit - 10);
} else {
cout << digit;
}
}
int main() {
int n, base;
cin >> n >> base;
printInt(n, base);
return 0;
}
```
函数的思路是将 n 不断除以 base,直到商小于 base 为止。然后依次输出每一位的余数,如果余数大于等于 10,输出相应的字母。如果 n 是负数,先输出负号,然后将 n 取反,继续进行上述操作即可。
您可以用以下格式调用该函数:
```c++
printInt(n, base);
```
其中 n 是待转换的数,base 是目标进制。
相关问题
首先编写一个函数 void printInt(int n, int base), 功能是输出 n 的 base 进制表示。 然后在以下主程序中调用。 int main(){ int x; cout << “请输入一个要转换的整数:”<< cout << “请输入一个要转换的整数:”<< endl; cin >>x; printInt(x, 2) ; printInt(x, 8) ; printInt(x, 16) ; return 0; }
好的,下面是实现 printInt 函数的代码:
```
#include <iostream>
using namespace std;
void printInt(int n, int base) {
if (n < 0) {
cout << "-";
n = -n;
}
if (n == 0) {
cout << "0";
return;
}
char digits[16] = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F'};
char result[32];
int i = 0;
while (n > 0) {
result[i++] = digits[n % base];
n /= base;
}
for (int j = i - 1; j >= 0; j--) {
cout << result[j];
}
cout << endl;
}
int main() {
int x;
cout << "请输入一个要转换的整数:" << endl;
cin >> x;
printInt(x, 2);
printInt(x, 8);
printInt(x, 16);
return 0;
}
```
这个函数的实现思路比较简单,就是不断地取余和除以 base,得到每一位的数字,并且将其存储在一个 char 数组中。最后再倒序输出这个数组即可。需要注意的是,当 n 小于 0 时需要先输出一个负号,并将 n 变成正数。同时,当 n 等于 0 时需要特判输出 0。
/给出下面程序输出结果。 #include <iostream> using namespace std; class Base { private: int Y; public: Base(int y = 0) { Y = y; cout << "Base(" << y << ")\n"; } ~Base() { cout << "~Base()\n"; } void print() { cout << Y << ""; } }; class Derived :public Base { private: int Z; public: Derived(int y, int z) :Base(y) { Z = z; cout << "Derived(" << y << "," << z << ")\n"; } ~Derived() { cout << "~Derived()\n"; } void print() { Base::print(); cout << Z << endl; } }; void main() { Derived d(10, 20); d.print(); }
程序输出结果为:
Base(10)
Derived(10,20)
10 20
其中,首先会调用 Derived 类的构造函数,该构造函数调用了 Base 类的构造函数,因此会输出 "Base(10)" 和 "Derived(10,20)"。接着调用 d.print() 函数,该函数先调用 Base 类的 print() 函数输出 Y 的值,再输出 Z 的值,因此最终的输出结果为 "10 20"。最后退出程序时会调用 Derived 类的析构函数和 Base 类的析构函数,分别输出 "~Derived()" 和 "~Base()"。
阅读全文
相关推荐
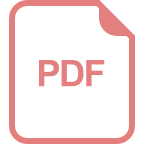














