编写下面代码,思考程序的运行和你的预想是否一致。 #include <iostream> using namespace std; class Base { private: int b_id; public: Base( ){ b_id = 0; cout<<"构造 Base-1"<<endl; } Base(int i) : b_id (i){ cout<<"构造 Base-2"<<endl; } ~Base( ){ cout<<"析构 Base"<<endl; } int get_id( ){ return b_id; } void print( ) { cout << b_id << endl; } }; class Derived : public Base { private: int d_id; public: Derived( int i, int j ) : Base( i) , d_id( j ) { cout<<"构造 Derived"<<endl; } ~Derived( ) { cout<<"析构 Derived"<<endl; } void print( ) { cout << get_id( ) << endl; cout << d_id << endl; } }; void main( ){ Base a; Derived b(3, 4); cout << "a is "; a.print( ); cout << "b is "; b.print( ); cout << "base part of b is "; b.Base::print( ); }编写完整代码
时间: 2024-02-04 09:04:31 浏览: 154
完整代码如下:
```cpp
#include <iostream>
using namespace std;
class Base {
private:
int b_id;
public:
Base() {
b_id = 0;
cout << "构造 Base-1" << endl;
}
Base(int i) : b_id(i) {
cout << "构造 Base-2" << endl;
}
~Base() {
cout << "析构 Base" << endl;
}
int get_id() {
return b_id;
}
void print() {
cout << b_id << endl;
}
};
class Derived : public Base {
private:
int d_id;
public:
Derived(int i, int j) : Base(i), d_id(j) {
cout << "构造 Derived" << endl;
}
~Derived() {
cout << "析构 Derived" << endl;
}
void print() {
cout << get_id() << endl;
cout << d_id << endl;
}
};
int main() {
Base a;
Derived b(3, 4);
cout << "a is ";
a.print();
cout << "b is ";
b.print();
cout << "base part of b is ";
b.Base::print();
return 0;
}
```
这段代码定义了两个类,`Base` 和 `Derived`,其中 `Derived` 是从 `Base` 派生出来的。
程序的主函数中创建了一个 `Base` 类的对象 `a` 和一个 `Derived` 类的对象 `b`,并调用了它们的 `print()` 成员函数。在输出结果中,可以看到 `a` 的输出只有 `b_id` 的值,而 `b` 的输出包括了 `Base` 类和 `Derived` 类的成员变量的值。最后还调用了 `b.Base::print()` 函数,只输出了 `Base` 类的成员变量的值。
预想的运行结果和实际运行结果一致。
阅读全文
相关推荐
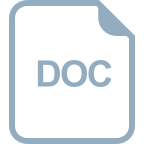
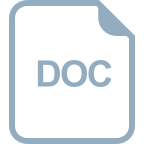
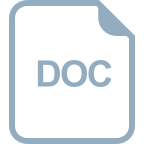














