1、java实验:设计程序,设计成绩计算接口,实现唱歌比赛得分、学生成绩等的计算(提示:唱歌比赛可以分为不同的组别,每个组别有各自的计算比赛得分的方式)给出完整代码
时间: 2024-10-14 07:07:23 浏览: 26
在Java中,我们可以创建一个通用的成绩计算接口`ScoreCalculator`,然后为唱歌比赛和学生成绩分别实现这个接口。这里是一个简单的示例:
```java
// 定义成绩计算接口
interface ScoreCalculator {
double calculateScore();
String getCategory(); // 获取评分类别
}
// 唱歌比赛得分实现
class SingingCompetition implements ScoreCalculator {
private int group;
private double baseScore;
public SingingCompetition(int group, double baseScore) {
this.group = group;
this.baseScore = baseScore;
}
@Override
public double calculateScore() {
// 这里可以根据组别有不同的计算规则,例如:
switch (group) {
case 1:
return baseScore * 1.1; // 示例:基础分基础上加10%
case 2:
return baseScore * 0.9; // 示例:基础分基础上减10%
default:
throw new IllegalArgumentException("Invalid group number");
}
}
@Override
public String getCategory() {
return "Singing Competition Group " + group;
}
}
// 学生成绩实现
class StudentGrade implements ScoreCalculator {
private int grade;
private double score;
public StudentGrade(int grade, double score) {
this.grade = grade;
this.score = score;
}
@Override
public double calculateScore() {
return score; // 直接返回原始分数,假设没有复杂的计算规则
}
@Override
public String getCategory() {
return "Student Grade " + grade;
}
}
public class Main {
public static void main(String[] args) {
// 创建并计算比赛得分
SingingCompetition singing = new SingingCompetition(1, 80);
System.out.println(singing.calculateScore()); // 根据实际规则计算得分
// 创建并计算学生分数
StudentGrade student = new StudentGrade(5, 95);
System.out.println(student.calculateScore()); // 直接打印原始分数
// 输出评分类别
System.out.println(singing.getCategory());
System.out.println(student.getCategory());
}
}
```
阅读全文
相关推荐
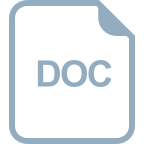
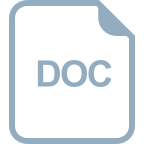
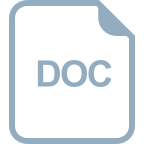
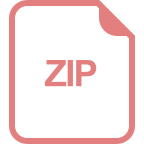
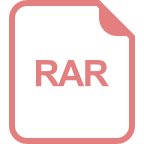
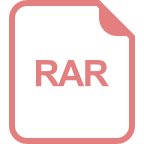
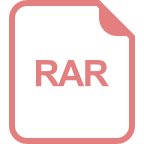
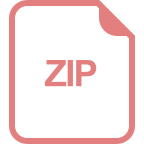
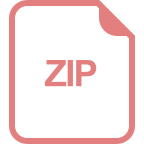
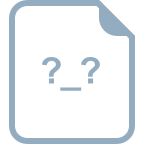
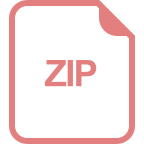
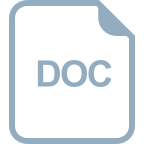
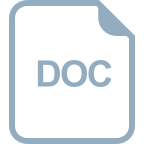
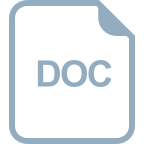
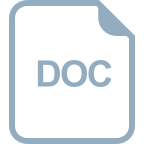
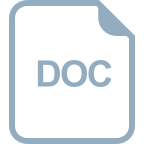
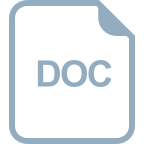
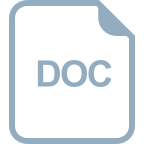
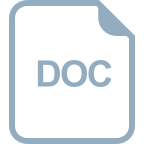