该程序完成从键盘输入一个字符串,将字符串中的大写字母转换成小写字母后进行输出的功能。C语言
时间: 2023-12-14 12:37:05 浏览: 31
可以使用C语言的标准库函数`tolower()`来将大写字母转换成小写字母。以下是实现该功能的代码示例:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
int i = 0;
while (str[i] != '\0') {
if (isupper(str[i])) { // 判断是否为大写字母
str[i] = tolower(str[i]); // 转换为小写字母
}
i++;
}
printf("转换后的字符串为:%s", str);
return 0;
}
```
在上面的代码中,首先定义一个长度为100的字符数组`str`,并通过`scanf()`函数从键盘输入字符串。然后,使用`while`循环遍历字符串中的每个字符,并使用`isupper()`函数判断是否为大写字母,如果是,则使用`tolower()`函数将其转换为小写字母。最后,使用`printf()`函数输出转换后的字符串。
相关问题
该程序完成从键盘输入一个字符串,C语言将字符串中的大写字母转换成小写字母后进行输出的功能。
以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
int i = 0;
while (str[i] != '\0') {
str[i] = tolower(str[i]);
i++;
}
printf("转换后的字符串:%s", str);
return 0;
}
```
代码解释:
1. 首先定义一个字符数组 `str`,用于存储输入的字符串。
2. 使用 `fgets()` 函数从标准输入读取一行字符串,并将其存储到 `str` 中。这里使用 `sizeof(str)` 来指定读取的字符串长度,避免输入字符串过长导致缓冲区溢出。
3. 使用 `tolower()` 函数将 `str` 中的大写字母转换成小写字母。具体来说,该函数会将大写字母转换成相应的小写字母,而对于非字母字符则不做处理。
4. 使用一个循环遍历 `str` 中的每个字符,并调用 `tolower()` 函数进行转换。
5. 最后,输出转换后的字符串。注意,这里使用 `%s` 格式化字符数组的输出,因为 `str` 已经是以 `\0` 结尾的字符串了。
用C语言,该程序完成从键盘输入一个字符串,将字符串中的大写字母转换成小写字母后进行输出的功能。
以下是一个示例程序:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
printf("Enter a string: ");
fgets(str, 100, stdin); // 从键盘读入字符串
for (int i = 0; i < strlen(str); i++) {
if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] += 32; // 转换为小写字母
}
}
printf("Result: %s", str);
return 0;
}
```
程序首先提示用户输入一个字符串,然后使用 `fgets()` 函数从键盘读入字符串并存储到 `str` 数组中。接着使用一个循环遍历字符串中的每个字符,如果该字符是大写字母,则将其转换为小写字母。最后输出转换后的字符串。
相关推荐
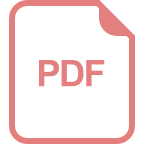
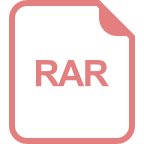












