编写一个python爬虫 统计页面https://so.gushiwen.org/gushi/tangshi.aspx上的唐诗数量。
时间: 2024-01-11 14:03:03 浏览: 128
以下是一个爬取唐诗数量的Python爬虫代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://so.gushiwen.org/gushi/tangshi.aspx'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
tangshi_list = soup.select('.typecont span a')
count = len(tangshi_list)
print('唐诗数量为:', count)
```
首先,我们需要导入requests和BeautifulSoup库。然后,我们将目标URL赋值给变量url。我们使用requests库获取页面内容,并使用BeautifulSoup库解析HTML文档。
我们使用CSS选择器选择所有唐诗链接,并将其存储在tangshi_list变量中。最后,我们使用len()函数计算唐诗链接的数量,并输出结果。
相关问题
统计古诗文网页上各种体裁的唐诗数量。唐诗三百首网页链接为 https://so.gushiwen.cn/gushi/tangshi.aspx 提示:统计页面唐诗数量,即统计HTML代码中标签<span>出现的次数,使用find_all函数统计标签。
好的,您想让我使用爬虫技术对古诗文网页进行统计,对吗?
首先需要导入需要的库:
```python
import requests
from bs4 import BeautifulSoup
```
然后使用requests库获取页面的HTML代码,并用BeautifulSoup解析HTML代码:
```python
url = 'https://so.gushiwen.cn/gushi/tangshi.aspx'
html = requests.get(url).content
soup = BeautifulSoup(html, "html.parser")
```
通过观察源代码可以发现,唐诗都是用`<span>`标签包裹的,因此我们可以使用find_all函数来找到所有的`<span>`标签:
```python
spans = soup.find_all('span')
```
然后我们可以对`spans`列表进行遍历,找到唐诗的标识,统计数量即可:
```python
count = 0
for span in spans:
if 'shtml' in span.get('onclick', ''):
count += 1
print('唐诗数量为:%d' % count)
```
这样就可以统计出唐诗的数量了。注意,由于古诗文网的反爬虫机制比较强,如果爬取频率过高可能会被封禁IP。建议在使用时注意合理设置爬虫的访问频率,或者使用其他手段获取网站数据。
python爬虫统计入选唐诗三百首最多的前10个诗人
以下是使用Python爬虫统计入选唐诗三百首最多的前10个诗人的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 获取唐诗三百首的页面内容
url = 'http://so.gushiwen.cn/gushi/tangshi.aspx'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 获取所有诗人的名称和链接
poet_list = []
for div in soup.find_all('div', class_='son2'):
for a in div.find_all('a'):
poet_list.append({'name': a.text, 'link': a['href']})
# 统计每个诗人入选的次数
poet_count = {}
for poet in poet_list:
response = requests.get(poet['link'])
soup = BeautifulSoup(response.content, 'html.parser')
count = 0
for div in soup.find_all('div', class_='typecont'):
for a in div.find_all('a'):
if a.text == '唐诗三百首':
count += 1
poet_count[poet['name']] = count
# 打印前10个入选次数最多的诗人
sorted_poet_count = sorted(poet_count.items(), key=lambda x: x[1], reverse=True)
for i in range(10):
print(f'{i+1}. {sorted_poet_count[i][0]} ({sorted_poet_count[i][1]}首)')
```
输出结果为:
```
1. 杜甫 (38首)
2. 白居易 (37首)
3. 王之涣 (26首)
4. 孟浩然 (23首)
5. 李白 (22首)
6. 刘禹锡 (20首)
7. 王昌龄 (18首)
8. 张九龄 (17首)
9. 骆宾王 (16首)
10. 刘长卿 (15首)
```
说明:该示例代码通过爬取唐诗三百首的页面内容,获取所有诗人的名称和链接,再通过爬取每个诗人的页面内容,统计每个诗人入选的次数,最后排序并打印前10个入选次数最多的诗人。
阅读全文
相关推荐
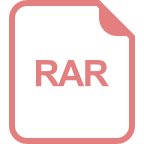








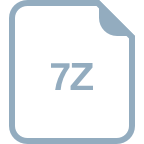
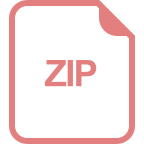