请帮我写一个通过openai的rest api进行图像处理的例子程序,使用go语言
时间: 2023-02-09 21:16:17 浏览: 67
。
Sure, here's a simple example of how you can use the OpenAI REST API for image processing in Go:
```
package main
import (
"bytes"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
func main() {
// Your OpenAI API key
apiKey := "YOUR_API_KEY"
// The URL for the OpenAI REST API endpoint for image processing
url := "https://api.openai.com/v1/images/generations"
// The image file to be processed
imageFile := "path/to/your/image.jpg"
// Read the image file into a byte slice
imageBytes, err := ioutil.ReadFile(imageFile)
if err != nil {
fmt.Println("Error reading image file:", err)
return
}
// Create a new HTTP client
client := &http.Client{}
// Create a new HTTP request
req, err := http.NewRequest("POST", url, bytes.NewBuffer(imageBytes))
if err != nil {
fmt.Println("Error creating HTTP request:", err)
return
}
// Set the API key as a header in the request
req.Header.Set("Authorization", "Bearer "+apiKey)
// Set the content type of the request to "image/jpeg"
req.Header.Set("Content-Type", "image/jpeg")
// Send the request
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error sending request:", err)
return
}
defer resp.Body.Close()
// Read the response body
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
// Unmarshal the response body into a Go struct
var responseData struct {
Data []struct {
ID string `json:"id"`
URL string `json:"url"`
} `json:"data"`
}
err = json.Unmarshal(body, &responseData)
if err != nil {
fmt.Println("Error unmarshaling response body:", err)
return
}
// Print the ID and URL of the processed image
for _, image := range responseData.Data {
fmt.Println("ID:", image.ID)
fmt.Println("URL:", image.URL)
}
}
```
This example uses the `ioutil` package to read the image file into a byte slice, the `net/http` package to send the request and receive the response, and the `encoding/json` package to unmarshal the response body into a Go struct.
Replace `YOUR_API_KEY` with your actual OpenAI API key, and replace `path/to/your/image.jpg` with the path to the image file you want to process.
I hope this helps! Let me know if you have any questions.
相关推荐
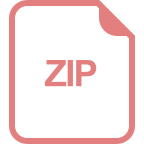
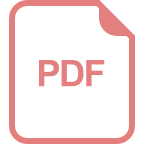
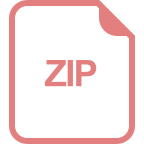














