双目摄像机标定python—opencv
时间: 2024-01-24 17:00:21 浏览: 33
双目摄像机标定是指通过对双目摄像机进行一系列的标定操作,来获取摄像机的内参和外参,从而实现双目摄像机的三维重建、立体匹配和立体测量等功能。
在Python中,可以使用OpenCV库来进行双目摄像机标定。首先需要安装OpenCV库,然后导入需要的模块。接着,通过一系列的标定图像对摄像机进行标定,获取摄像机的内参和外参。这个过程通常包括拍摄一组棋盘格图像,并使用这些图像来计算摄像机的标定参数。
在使用OpenCV进行双目摄像机标定时,可以使用函数cv2.stereoCalibrate()来计算摄像机的内参和外参,函数cv2.stereoRectify()来对摄像机进行校正,以提高三维重建和立体匹配的精度。同时还可以使用函数cv2.initUndistortRectifyMap()和cv2.remap()来将校正后的图像映射到新的坐标系中。
在完成标定和校正之后,就可以利用双目摄像机进行三维重建、立体匹配和立体测量等功能。例如,可以使用双目摄像机来获取场景中物体的三维坐标,或者进行深度测量和立体视觉跟踪等应用。
总之,通过Python和OpenCV库,可以方便地对双目摄像机进行标定和校正,为后续的三维重建和立体匹配等应用提供重要的基础支持。
相关问题
摄像机标定python
要进行摄像机标定,需要使用OpenCV库中的cv2.calibrateCamera()函数。这个函数需要至少10个标定图像,每个标定图像中至少有6个已知的3D物体点和它们在图像中对应的2D图像点。以下是一个基本的Python代码示例,用于进行摄像机标定:
```
import numpy as np
import cv2
# 定义标定板的尺寸
board_size = (7, 7)
# 准备3D物体点的空间坐标
objp = np.zeros((board_size[0]*board_size[1], 3), np.float32)
objp[:,:2] = np.mgrid[0:board_size[0],0:board_size[1]].T.reshape(-1,2)
# 存储物体点和图像点的坐标
obj_points = [] # 3D物体点
img_points = [] # 2D图像点
# 获取标定图像
images = glob.glob('calibration_images/*.jpg')
# 循环处理每张标定图像
for fname in images:
img = cv2.imread(fname)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 寻找棋盘格角点
ret, corners = cv2.findChessboardCorners(gray, board_size, None)
# 如果成功找到角点,将物体点和图像点添加到列表中
if ret == True:
obj_points.append(objp)
img_points.append(corners)
# 在图像上绘制角点
cv2.drawChessboardCorners(img, board_size, corners, ret)
cv2.imshow('img', img)
cv2.waitKey(500)
cv2.destroyAllWindows()
# 进行相机标定
ret, mtx, dist, rvecs, tvecs = cv2.calibrateCamera(obj_points, img_points, gray.shape[::-1], None, None)
# 输出相机内参和畸变系数
print("Camera Matrix:\n", mtx)
print("\nDistortion Coefficients:\n", dist)
```
这个代码示例假设标定板的尺寸为7x7,标定图像存储在名为“calibration_images”的文件夹中。它将尝试找到每个标定图像中的棋盘格角点,并将它们添加到物体点和图像点列表中。最后,它将使用这些点来进行相机标定,并输出相机内参和畸变系数。
python opencv 自标定
自标定(self-calibration)是指在相机标定的过程中,利用摄像机自身的运动和观察到的图像特征,来估计相机的内外参数,从而减少或消除标定时所需的专用标定图像。
在Python和OpenCV中,可以使用自标定方法来估计相机参数。下面是一个基本的自标定步骤:
1. 收集不同角度和位置下的图像序列,要求至少包含10-20张图像。
2. 通过OpenCV库中的图片读取函数读取图像序列。
3. 在图像中提取特征点,可以使用OpenCV中的ORB、SIFT或SURF等特征提取算法。
4. 将特征点匹配到一起,可以使用OpenCV中的FLANN或BFMatcher算法进行特征点匹配。
5. 使用RANSAC算法从匹配的特征点中估计基础矩阵或本质矩阵,并恢复相机的内外参数。
6. 对相机内外参数进行优化,可以使用Levenberg-Marquardt等非线性优化算法。
7. 重复步骤3-6直到收敛。
注意事项:
- 图像序列应该尽可能包含不同的角度、位置和运动轨迹,以保证标定结果的准确性。
- 特征提取和匹配算法的选择应根据具体情况进行调整,以获得最佳的特征匹配效果。
- 在标定过程中,需要对相机的焦距、畸变等参数进行估计和优化,以获得更准确的标定结果。
总之,Python和OpenCV提供了丰富的图像处理和计算机视觉功能,可以实现相机的自标定。自标定方法可以极大地简化相机标定的过程,并且减少了对专用标定图像的依赖。
相关推荐
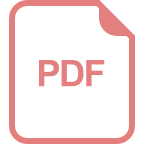
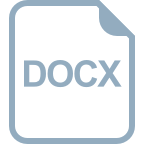
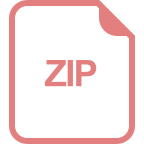












