cat(): argument 'tensors' (position 1) must be tuple of Tensors, not Tensor
时间: 2024-10-01 16:11:00 浏览: 81
这个错误提示来自PyTorch库,当你试图调用`cat()`函数时,它期望接收的是一个元组(tuple)作为第一个参数,其中包含多个张量(Tensors)。但实际上,你传递给它的是一个单独的张量(Tensor)。`cat()`用于沿着指定维度将多个张量串联成一个大张量。
例如,正确的用法应该是这样的:
```python
tensor_list = [tensor1, tensor2, tensor3] # 这里tensor1, tensor2, tensor3都是Tensors
result = torch.cat(tensor_list, dim=dim) # dim是你想要合并的维度,可以是0, 1, 2等
```
如果你想合并一个张量和另一个张量,应该先将它们放入一个元组中:
```python
single_tensor = some_tensor # single_tensor是一个张量
result = torch.cat((single_tensor, another_tensor), dim=dim)
```
如果你的错误是因为某个地方直接使用了`cat(some_tensor)`而不是`(some_tensor,)`,那么你需要检查并修正那个部分的代码。
相关问题
TypeError: stack(): argument 'tensors' (position 1) must be tuple of Tensors, not Tensor
This error occurs when the `stack()` function in PyTorch is called with a single tensor instead of a tuple of tensors.
To resolve this error, you need to pass a tuple of tensors to the `stack()` function instead of a single tensor. For example:
```
import torch
# Create two tensors
tensor1 = torch.tensor([1, 2, 3])
tensor2 = torch.tensor([4, 5, 6])
# Use stack() function to stack the tensors
stacked_tensors = torch.stack((tensor1, tensor2))
print(stacked_tensors)
```
This will output:
```
tensor([[1, 2, 3],
[4, 5, 6]])
```
Notice that we passed a tuple of tensors `(tensor1, tensor2)` to the `stack()` function.
TypeError: conv2d(): argument 'padding' must be tuple of ints, not float
这个错误是因为conv2d()函数的padding参数要求是一个整数元组(tuple of ints),而不是一个浮点数。你需要将window_size/2转换为整数,并将其作为padding参数的值。
你可以使用int()函数将浮点数转换为整数。在调用conv2d()函数时,将padding参数的值设置为一个包含window_size/2的整数元组。
例如,你可以这样修改代码:
```python
mu1 = F.conv2d(img1_tensor, window, padding=(int(window_size/2), int(window_size/2)), groups=channel)
```
这样应该能解决这个TypeError。确保其他相关变量也进行相应的类型转换。
阅读全文
相关推荐
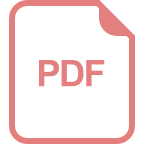
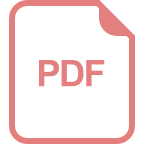














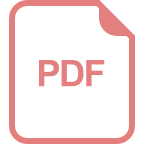