多项式求导函数c语言
时间: 2024-12-12 12:14:37 浏览: 13
在C语言中,计算多项式的导数通常涉及到对每个项应用幂规则(即对于形如f(x) = ax^n,其导数f'(x) = n*ax^(n-1))。为了编写这样一个函数,你可以创建一个函数库,其中包含一个通用的函数来处理单个项的导数,然后递归地应用于整个多项式。
下面是一个简单的示例,假设我们有一个多项式数组`coeffs`存储了多项式的系数(从常数项到最高次项),`degree`表示多项式的次数:
```c
#include <stdio.h>
#include <stdlib.h>
// 计算单个项的导数
double derivative(double x, int coeff, int power) {
return coeff * power * pow(x, power - 1);
}
// 多项式导数函数
double polynomial_derivative(const double *coeffs, int degree) {
if (degree == 0) // 基本情况,如果多项式是一次或常数项,则导数不变
return coeffs[0];
double result = 0;
for (int i = 1; i <= degree; ++i)
result += derivative(coeffs[i], i, degree); // 逐项累加
return result;
}
int main() {
double *coeffs = malloc(degree * sizeof(double)); // 假设已填充多项式系数
int degree = ...; // 实际多项式的次数
double derivative_value = polynomial_derivative(coeffs, degree);
printf("多项式 %s 的导数为 %.2f\n", "... (填写多项式表达式)", derivative_value);
free(coeffs);
return 0;
}
```
记得在实际使用时,需要处理输入错误(如`coeffs`为空、非零除数等)并确保`degree`为正整数。此外,在`main()`函数中,你需要提供具体的多项式表达式和系数。
阅读全文
相关推荐
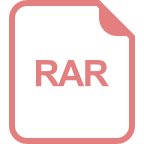
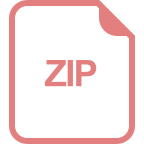
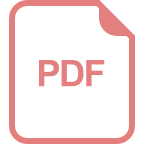



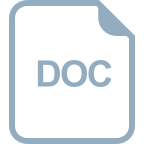
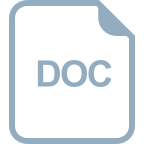

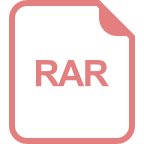





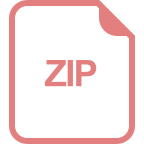
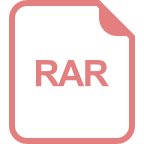
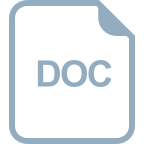
