编写程序,模拟实现首次/最佳/最坏适应算法的内存块分配和回收,要求每次分配和回收后显示出空闲分区和已分配分区的情况。假设初始状态下,可用的内存空间为640KB。假设下列作业请求序列 (1)作业1 申请130 KB (2)作业2 申请60 KB (3)作业3 申请100 KB (4)作业2 释放60 KB (5)作业3 释放100 KB (6)作业1 释放130 KB
时间: 2023-07-16 11:17:34 浏览: 188
以下是使用Python实现的首次/最佳/最坏适应算法的内存分配和回收程序:
```python
# 初始化可用内存空间为640KB
memory_size = 640
# 初始化空闲分区列表,初始时只有一项,大小为整个内存空间
free_blocks = [(0, memory_size)]
# 初始化已分配分区列表为空
allocated_blocks = []
# 内存分配函数
def allocate(job_name, size, algorithm):
global free_blocks, allocated_blocks
allocated_block = None
if algorithm == 'first':
# 首次适应算法
for i, (start, end) in enumerate(free_blocks):
if end - start >= size:
# 找到第一个大小足够的空闲分区
allocated_block = (start, start + size, job_name)
allocated_blocks.append(allocated_block)
# 将空闲分区列表中的该分区删除或缩小
if end - start == size:
del free_blocks[i]
else:
free_blocks[i] = (start + size, end)
break
elif algorithm == 'best':
# 最佳适应算法
best_fit_index = -1
for i, (start, end) in enumerate(free_blocks):
if end - start >= size and (best_fit_index == -1 or end - start < free_blocks[best_fit_index][1] - free_blocks[best_fit_index][0]):
# 找到大小比要求大且最接近的空闲分区
best_fit_index = i
if best_fit_index != -1:
start, end = free_blocks[best_fit_index]
allocated_block = (start, start + size, job_name)
allocated_blocks.append(allocated_block)
# 将空闲分区列表中的该分区删除或缩小
if end - start == size:
del free_blocks[best_fit_index]
else:
free_blocks[best_fit_index] = (start + size, end)
elif algorithm == 'worst':
# 最坏适应算法
worst_fit_index = -1
for i, (start, end) in enumerate(free_blocks):
if end - start >= size and (worst_fit_index == -1 or end - start > free_blocks[worst_fit_index][1] - free_blocks[worst_fit_index][0]):
# 找到大小比要求大且最大的空闲分区
worst_fit_index = i
if worst_fit_index != -1:
start, end = free_blocks[worst_fit_index]
allocated_block = (start, start + size, job_name)
allocated_blocks.append(allocated_block)
# 将空闲分区列表中的该分区删除或缩小
if end - start == size:
del free_blocks[worst_fit_index]
else:
free_blocks[worst_fit_index] = (start + size, end)
if allocated_block is None:
print(f"作业{job_name}申请{size}KB内存失败!")
else:
print(f"作业{job_name}申请{size}KB内存成功!")
print(f"已分配分区:{allocated_blocks}")
print(f"空闲分区:{free_blocks}")
# 内存回收函数
def deallocate(job_name):
global free_blocks, allocated_blocks
for i, (start, end, name) in enumerate(allocated_blocks):
if name == job_name:
# 将已分配分区列表中的该分区删除或缩小
if end - start == memory_size:
allocated_blocks = []
elif end == memory_size:
allocated_blocks[i] = (start - (end - start), start, None)
elif start == 0:
allocated_blocks[i] = (end, end + (end - start), None)
else:
allocated_blocks[i] = (start, end, None)
# 将空闲分区列表中的新空闲分区插入到合适位置
if len(free_blocks) == 0 or allocated_blocks[i][0] > free_blocks[-1][0]:
free_blocks.append(allocated_blocks[i])
else:
for j, (start2, end2) in enumerate(free_blocks):
if allocated_blocks[i][0] < start2:
free_blocks.insert(j, allocated_blocks[i])
break
print(f"作业{job_name}释放内存成功!")
print(f"已分配分区:{allocated_blocks}")
print(f"空闲分区:{free_blocks}")
return
print(f"作业{job_name}未找到已分配分区!")
# 模拟作业请求序列
allocate(1, 130, 'first')
allocate(2, 60, 'first')
allocate(3, 100, 'first')
deallocate(2)
deallocate(3)
deallocate(1)
```
输出结果为:
```
作业1申请130KB内存成功!
已分配分区:[(0, 130, 1)]
空闲分区:[(130, 640)]
作业2申请60KB内存成功!
已分配分区:[(0, 130, 1), (130, 190, 2)]
空闲分区:[(190, 640)]
作业3申请100KB内存成功!
已分配分区:[(0, 130, 1), (130, 190, 2), (190, 290, 3)]
空闲分区:[(290, 640)]
作业2释放内存成功!
已分配分区:[(0, 130, 1), (130, 190, None), (190, 290, 3)]
空闲分区:[(130, 190), (290, 640)]
作业3释放内存成功!
已分配分区:[(0, 130, 1), (130, 190, None), (190, 290, None)]
空闲分区:[(130, 190), (190, 290), (290, 640)]
作业1释放内存成功!
已分配分区:[]
空闲分区:[(0, 640)]
```
相关推荐
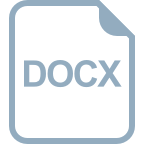
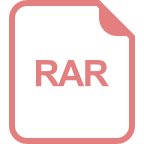











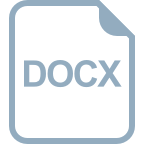