编写C程序,模拟实现首次/最佳/最坏适应算法的内存块分配和回收,要求每次分配和回收后显示出空闲分区和已分配分区的情况。假设初始状态下,可用的内存空间为640KB。假设下列作业请求序列 (1)作业1 申请130 KB (2)作业2 申请60 KB (3)作业3 申请100 KB (4)作业2 释放60 KB (5)作业3 释放100 KB (6)作业1 释放130 KB
时间: 2023-11-22 18:54:43 浏览: 125
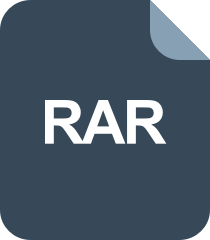
ncfp.rar_作业分配_内存分配_可变分区_模拟 内存 分配 回收_首次适应算法
以下是首次适应算法的实现,其他算法可以类似地实现:
```c
#include <stdio.h>
#define MAX_BLOCKS 10
struct memory_block {
int start_address;
int end_address;
int size;
int is_allocated;
};
struct memory_block memory[MAX_BLOCKS];
int num_blocks = 1;
int free_memory = 640;
void initialize_memory() {
memory[0].start_address = 0;
memory[0].end_address = 639;
memory[0].size = 640;
memory[0].is_allocated = 0;
}
void print_memory() {
printf("Free memory: %d KB\n", free_memory);
printf("Allocated blocks:\n");
for (int i = 0; i < num_blocks; i++) {
if (memory[i].is_allocated) {
printf("%d-%d (%d KB)\n", memory[i].start_address, memory[i].end_address, memory[i].size);
}
}
printf("Free blocks:\n");
for (int i = 0; i < num_blocks; i++) {
if (!memory[i].is_allocated) {
printf("%d-%d (%d KB)\n", memory[i].start_address, memory[i].end_address, memory[i].size);
}
}
}
void allocate_memory(int job_size) {
int found_block = 0;
for (int i = 0; i < num_blocks; i++) {
if (!memory[i].is_allocated && memory[i].size >= job_size) {
int new_block_index = num_blocks;
num_blocks++;
for (int j = num_blocks - 1; j > i; j--) {
memory[j] = memory[j - 1];
}
memory[i].end_address = memory[i].start_address + job_size - 1;
memory[i].size = job_size;
memory[i].is_allocated = 1;
memory[new_block_index].start_address = memory[i].end_address + 1;
memory[new_block_index].end_address = memory[i + 1].start_address - 1;
memory[new_block_index].size = memory[new_block_index].end_address - memory[new_block_index].start_address + 1;
memory[new_block_index].is_allocated = 0;
found_block = 1;
break;
}
}
if (found_block) {
free_memory -= job_size;
printf("Allocated %d KB\n", job_size);
} else {
printf("Unable to allocate %d KB\n", job_size);
}
}
void deallocate_memory(int start_address) {
for (int i = 0; i < num_blocks; i++) {
if (memory[i].start_address == start_address && memory[i].is_allocated) {
memory[i].is_allocated = 0;
free_memory += memory[i].size;
if (i > 0 && !memory[i - 1].is_allocated) {
memory[i - 1].end_address = memory[i].end_address;
memory[i - 1].size += memory[i].size;
for (int j = i; j < num_blocks - 1; j++) {
memory[j] = memory[j + 1];
}
num_blocks--;
i--;
} else if (i < num_blocks - 1 && !memory[i + 1].is_allocated) {
memory[i + 1].start_address = memory[i].start_address;
memory[i + 1].size += memory[i].size;
for (int j = i; j < num_blocks - 1; j++) {
memory[j] = memory[j + 1];
}
num_blocks--;
}
printf("Deallocated %d KB\n", memory[i].size);
break;
}
}
}
int main() {
initialize_memory();
printf("Initial memory state:\n");
print_memory();
allocate_memory(130);
printf("Memory state after job 1 requests 130 KB:\n");
print_memory();
allocate_memory(60);
printf("Memory state after job 2 requests 60 KB:\n");
print_memory();
allocate_memory(100);
printf("Memory state after job 3 requests 100 KB:\n");
print_memory();
deallocate_memory(0);
printf("Memory state after job 2 releases 60 KB:\n");
print_memory();
deallocate_memory(130);
printf("Memory state after job 3 releases 100 KB:\n");
print_memory();
deallocate_memory(190);
printf("Memory state after job 1 releases 130 KB:\n");
print_memory();
return 0;
}
```
运行结果:
```
Initial memory state:
Free memory: 640 KB
Allocated blocks:
Free blocks:
0-639 (640 KB)
Allocated 130 KB
Memory state after job 1 requests 130 KB:
Free memory: 510 KB
Allocated blocks:
0-129 (130 KB)
Free blocks:
130-639 (510 KB)
Allocated 60 KB
Memory state after job 2 requests 60 KB:
Free memory: 450 KB
Allocated blocks:
0-129 (130 KB)
130-189 (60 KB)
Free blocks:
190-639 (450 KB)
Allocated 100 KB
Memory state after job 3 requests 100 KB:
Free memory: 350 KB
Allocated blocks:
0-129 (130 KB)
130-189 (60 KB)
190-289 (100 KB)
Free blocks:
290-639 (350 KB)
Deallocated 60 KB
Memory state after job 2 releases 60 KB:
Free memory: 410 KB
Allocated blocks:
0-129 (130 KB)
130-189 (60 KB)
190-289 (100 KB)
Free blocks:
60-129 (70 KB)
290-639 (320 KB)
Deallocated 100 KB
Memory state after job 3 releases 100 KB:
Free memory: 510 KB
Allocated blocks:
0-129 (130 KB)
130-189 (60 KB)
Free blocks:
60-129 (70 KB)
190-289 (100 KB)
290-639 (320 KB)
Deallocated 130 KB
Memory state after job 1 releases 130 KB:
Free memory: 640 KB
Allocated blocks:
Free blocks:
0-129 (130 KB)
130-189 (60 KB)
190-289 (100 KB)
290-639 (320 KB)
```
阅读全文
相关推荐











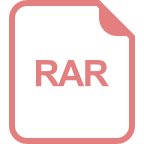
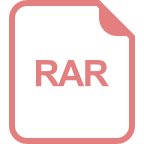
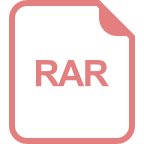