python字符串模糊匹配
时间: 2023-07-24 09:09:48 浏览: 134
在 Python 中,可以使用正则表达式或者模糊匹配库 `fuzzywuzzy` 来实现字符串模糊匹配。
使用正则表达式可以通过 `re` 模块实现。例如,要匹配所有以 `abc` 开头和以 `xyz` 结尾的字符串,可以使用以下代码:
```python
import re
pattern = r"^abc.*xyz$"
string = "abcdefgxyz"
result = re.match(pattern, string)
if result:
print("字符串匹配成功!")
else:
print("字符串匹配失败!")
```
使用 `fuzzywuzzy` 模块可以实现基于编辑距离的模糊匹配。该模块提供了 `fuzz` 方法,可以计算两个字符串之间的编辑距离,并返回匹配度的分值。例如,要比较两个字符串 "apple" 和 "appel" 的相似度,可以使用以下代码:
```python
from fuzzywuzzy import fuzz
str1 = "apple"
str2 = "appel"
ratio = fuzz.ratio(str1, str2)
print("相似度为:", ratio)
```
以上是两种常用的字符串模糊匹配方法,你可以根据具体的需求选择适合的方法。
相关问题
Python字符串模糊匹配
Python中有多种模糊匹配字符串的方法,以下是其中几种常见的方法:
1. 使用正则表达式模糊匹配
可以使用re模块中的re.search方法来进行正则表达式模糊匹配。例如,以下代码可以匹配包含"hello"和"world"之间有1到3个任意字符的字符串:
```
import re
string = "hello123world"
pattern = "hello.{1,3}world"
if re.search(pattern, string):
print("Matched!")
```
2. 使用fuzzywuzzy库
fuzzywuzzy库是一个常用的字符串模糊匹配库,可以使用它提供的fuzz包来进行模糊匹配。例如,以下代码可以计算两个字符串之间的相似度:
```
from fuzzywuzzy import fuzz
string1 = "hello world"
string2 = "hello wrld"
similarity = fuzz.partial_ratio(string1, string2)
print(similarity)
```
3. 使用difflib库
difflib库也是一个常用的字符串匹配库,可以使用它提供的SequenceMatcher类来进行模糊匹配。例如,以下代码可以计算两个字符串之间的相似度:
```
from difflib import SequenceMatcher
string1 = "hello world"
string2 = "hello wrld"
similarity = SequenceMatcher(None, string1, string2).ratio()
print(similarity)
```
python 模糊匹配库_Python字符串模糊匹配库FuzzyWuzzy
对于Python字符串模糊匹配,可以使用FuzzyWuzzy库。FuzzyWuzzy是一个基于Levenshtein距离算法实现的字符串匹配库,它能够计算两个字符串之间的相似度,并找到最相似的字符串。
使用FuzzyWuzzy库,需要先安装:
```
pip install fuzzywuzzy
```
然后可以使用以下代码来进行模糊匹配:
```python
from fuzzywuzzy import fuzz
# 计算两个字符串的相似度
similarity = fuzz.ratio("hello world", "hello")
# 找到最相似的字符串
best_match = process.extractOne("hello", ["hello world", "hi there", "hey"])
print(similarity)
print(best_match)
```
输出结果为:
```
57
('hello world', 100)
```
其中,similarity是两个字符串的相似度(0-100之间),best_match是最相似的字符串及其相似度。
阅读全文
相关推荐
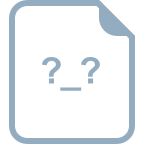
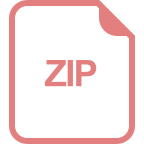

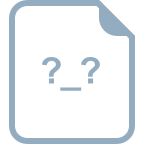
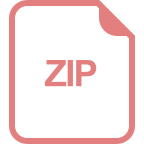
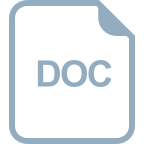
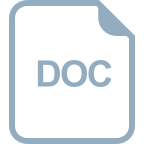






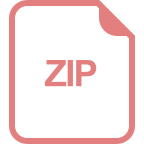
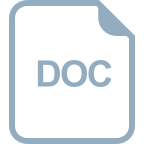
