用顺序队的方式写 不用next
时间: 2024-10-16 20:14:33 浏览: 15
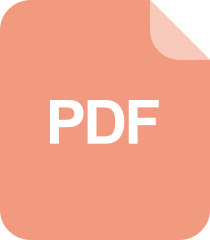
JS多个异步请求 按顺序执行next实现解析

如果你不想使用链表(通过 `next` 指针连接队列元素),而是选择顺序存储所有元素,那么你需要改变数据结构和插入/删除操作。在这种情况下,你可以使用数组来实现队列,但是进出队操作会变得复杂,因为当你满队或出队时需要移动数组中的元素。
以下是使用顺序队列(数组实现)的基本结构和操作:
```c
#define MAXSIZE 10
#include<stdio.h>
#include<stdlib.h>
typedef struct {
char data[MAXSIZE];
int front, rear; // 使用两个整数表示队首(front)和队尾(rear)
} SeQueue;
// 初始化顺序队列
SeQueue *init_Queue() {
SeQueue *q = (SeQueue*) malloc(sizeof(SeQueue));
q->front = -1;
q->rear = -1;
return q;
}
// 数据元素x进队(当队尾未达到最大容量时)
void Enqueue(SeQueue *q, char x) {
if (q->rear + 1 >= MAXSIZE) {
printf("Queue is full, cannot enqueue.\n");
return;
}
q->rear++;
q->data[q->rear] = x;
}
// 数据元素出队(从队首取出并更新队首位置)
char Dequeue(SeQueue *q) {
if (q->front == q->rear) {
printf("Queue is empty, dequeue operation not allowed.\n");
return '\0';
}
char x = q->data[q->front];
q->front++;
return x;
}
// 判断队列是否为空
int IsEmpty(SeQueue *q) {
return q->front == q->rear;
}
void main() {
SeQueue *q = init_Queue();
char x = 'e', y = 'c';
Enqueue(q, 'h');
Enqueue(q, 'r');
Enqueue(q, y);
char dequeued_x = Dequeue(q);
Enqueue(q, x);
char dequeued_y = Dequeue(q);
Enqueue(q, 'a');
while (!IsEmpty(q)) {
char removed_char = Dequeue(q);
printf("%c", removed_char);
}
printf("\n%d\n", x);
free(q); // 程序结束前记得释放队列内存
}
阅读全文
相关推荐
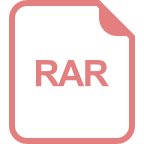
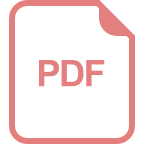
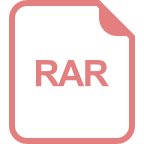
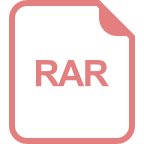
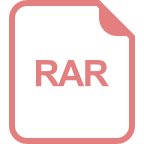
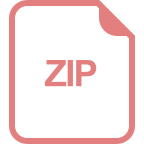










