使图像颜色均匀代码python
时间: 2023-10-14 17:27:34 浏览: 84
实现图像颜色均匀化的代码如下:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算直方图
hist = cv2.calcHist([gray], [0], None, [256], [0, 256])
# 计算累计分布函数
cdf = hist.cumsum()
# 归一化
cdf_normalized = cdf * hist.max() / cdf.max()
# 计算均衡化后的像素值
equalized = cv2.normalize(gray, None, 0, 255, cv2.NORM_MINMAX)
# 显示原始图像和均衡化后的图像
cv2.imshow('Original', gray)
cv2.imshow('Equalized', equalized)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码首先读取图像,然后将其转换为灰度图像。接着,使用 `cv2.calcHist()` 函数计算图像的直方图,然后计算累计分布函数。接下来,将累计分布函数归一化,以便将其作为像素值的映射表。最后,使用 `cv2.normalize()` 函数将图像进行均衡化,并显示原始图像和均衡化后的图像。
相关问题
灰度图像实现反差基本一致、相邻细部反差增大,颜色均匀,反差适中的算法python实现
可以使用以下代码实现:
```python
import cv2
import numpy as np
# 读取灰度图像
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 计算最大灰度值和最小灰度值
min_val, max_val, _, _ = cv2.minMaxLoc(img)
# 计算灰度级别
levels = 256
# 计算增益和偏移量
gain = (levels-1) / (max_val - min_val)
bias = -min_val * gain
# 计算反差增强后的图像
img_contrast = cv2.convertScaleAbs(img, alpha=gain, beta=bias)
# 显示原始图像和反差增强后的图像
cv2.imshow('Original Image', img)
cv2.imshow('Contrast Enhanced Image', img_contrast)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们首先读取了灰度图像,并计算了最大灰度值和最小灰度值。接下来,我们计算了灰度级别,并根据最大灰度值、最小灰度值、灰度级别计算了增益和偏移量。最后,我们使用cv2.convertScaleAbs函数对图像进行反差增强,并将原始图像和反差增强后的图像显示出来。
值得注意的是,上述代码中计算的增益和偏移量是基于整个图像的最大灰度值和最小灰度值计算的。如果需要对图像的局部区域进行反差增强,可以先对图像进行分割,然后分别计算每个局部区域的最大灰度值和最小灰度值,并基于这些值计算增益和偏移量。
图像预处理降噪增强对比度 代码
图像预处理是一个关键步骤,用于改善图像的质量、增强特征可读性并准备数据输入到机器学习模型中。降噪、增强对比度和预处理通常涉及到以下几个步骤:
1. **降噪** (Noise Reduction):
- 使用滤波器:例如高斯滤波器(gaussian blur)、中值滤波器(median filter)或小波去噪(wavelet denoising)来减少噪声。
- 基于统计的方法:比如均值滤波、自适应阈值方法(如Otsu's方法)。
```python
from skimage import filters
img = ... # 输入图像
denoised_img = filters.gaussian(img, sigma=1) # 使用高斯滤波器降噪
```
2. **增强对比度** (Contrast Enhancement):
- 对比度拉伸:通过线性变换将图像的像素值缩放,使图像细节更明显。
- 自适应直方图均衡化:如CLAHE (Contrast Limited Adaptive Histogram Equalization),局部区域均衡化避免全局均匀化导致的过度强化细节。
```python
from skimage.exposure import equalize_adapthist
img = ... # 输入图像
contrast_enhanced_img = equalize_adapthist(img)
```
3. **色彩空间转换** (Color Space Conversion):
- 有时可能需要从彩色转换到灰度(如二值化操作),或者调整颜色空间(RGB -> HSV/ LAB等)。
```python
from skimage.color import rgb2gray, lab2rgb
gray_img = rgb2gray(img)
```
4. **边缘检测和锐化** (Edge Detection & Sharpening):
- 使用Canny、Sobel或Prewitt算子进行边缘检测。
- 锐化操作可通过高斯卷积后减去原图像。
```python
from skimage.feature import canny
edge_img = canny(img)
img_sharpened = cv2.filter2D(img, -1, kernel=np.array([[-1, -1, -1], [-1, 9, -1], [-1, -1, -1]])) # 简单锐化
```
相关推荐
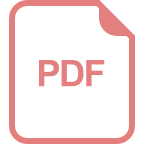
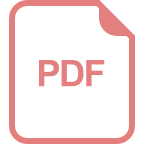
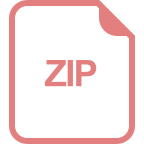
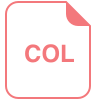











