用前后端分离写一个转账带事务注解(EnableTransactionManagement)
时间: 2023-11-07 13:09:32 浏览: 31
好的,下面是一个简单的前后端分离的转账应用的示例代码:
后端代码(使用Spring Boot框架和JPA):
```java
@RestController
@RequestMapping("/api/transfer")
@EnableTransactionManagement
public class TransferController {
@Autowired
private TransferRepository transferRepository;
@PostMapping
@Transactional
public ResponseEntity<?> transfer(@RequestBody TransferDTO transferDTO) {
TransferEntity from = transferRepository.findById(transferDTO.getFromAccountId()).orElseThrow(() -> new RuntimeException("Account not found"));
TransferEntity to = transferRepository.findById(transferDTO.getToAccountId()).orElseThrow(() -> new RuntimeException("Account not found"));
if (from.getBalance() < transferDTO.getAmount()) {
throw new RuntimeException("Insufficient balance");
}
from.setBalance(from.getBalance() - transferDTO.getAmount());
to.setBalance(to.getBalance() + transferDTO.getAmount());
transferRepository.save(from);
transferRepository.save(to);
return ResponseEntity.ok().build();
}
}
```
前端代码(使用Vue.js框架和Axios库):
```html
<template>
<div>
<form @submit.prevent="transfer">
<label>From Account:</label>
<input type="text" v-model="fromAccountId" required>
<br>
<label>To Account:</label>
<input type="text" v-model="toAccountId" required>
<br>
<label>Amount:</label>
<input type="text" v-model="amount" required>
<br>
<button type="submit">Transfer</button>
</form>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
fromAccountId: '',
toAccountId: '',
amount: ''
}
},
methods: {
transfer() {
axios.post('/api/transfer', {
fromAccountId: this.fromAccountId,
toAccountId: this.toAccountId,
amount: this.amount
}).then(() => {
alert('Transfer successful!')
}).catch(error => {
alert('Transfer failed: ' + error.response.data.message)
})
}
}
}
</script>
```
在这个例子中,我们使用了`@EnableTransactionManagement`注解来启用事务管理,同时使用了`@Transactional`注解来标记转账方法,以确保在转账过程中如果任何一个操作失败,整个事务会回滚。此外,我们还使用了JPA和Vue.js框架来实现数据持久化和前端UI。
相关推荐
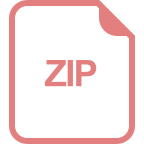
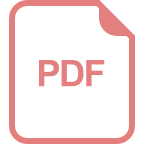














