编写程序实现以下功能:利用subplot绘制sin(2x)、2sin(2x)cos(x)、sin(x)/cos(x )的图形
时间: 2024-10-16 21:13:45 浏览: 26
在Python中,我们可以使用matplotlib库中的subplot函数来同时绘制多个相关的图形。下面是一个示例,演示如何编写程序实现这个功能:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义x值范围
x = np.linspace(0, 2 * np.pi, 400)
# 计算各个函数的值
y1 = np.sin(2*x)
y2 = 2*np.sin(2*x)*np.cos(x)
y3 = np.sin(x) / np.cos(x)
fig, axs = plt.subplots(nrows=2, ncols=2, figsize=(8, 6), sharex=True, sharey=True)
axs[0, 0].plot(x, y1, label='sin(2x)')
axs[0, 0].set_title('Subplot 1')
axs[0, 0].legend()
axs[0, 1].plot(x, y2, label='2sin(2x)cos(x)')
axs[0, 1].set_title('Subplot 2')
axs[1, 0].plot(x, y3, label='sin(x)/cos(x)')
axs[1, 0].set_title('Subplot 3')
# 对于可能的除零错误,我们添加了异常处理
try:
y4 = np.sin(x) / (np.cos(x) + 1e-9) # 避免分母接近0的问题
except RuntimeWarning:
print("Note: Division by zero avoided in subplot 4.")
if not np.isnan(y4).all(): # 如果没有发生nan,才画图
axs[1, 1].plot(x, y4, label='sin(x)/(cos(x)+epsilon)')
axs[1, 1].set_title('Subplot 4')
for ax in axs.flat:
ax.set(xlim=(0, 2*np.pi), ylim=(-2, 2))
ax.grid(True)
ax.legend()
plt.tight_layout() # 使子图之间有足够的间距
plt.show()
```
在这个例子中,我们创建了一个2行2列的网格布局,每个子图对应一个函数的曲线。注意在计算`sin(x)/cos(x)`时,我们加了一个极小的数值避免分母为零的警告。
阅读全文
相关推荐
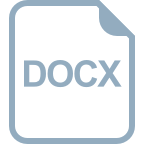
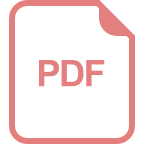
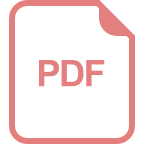
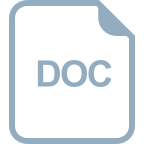
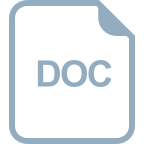
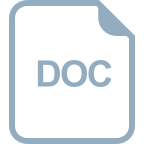
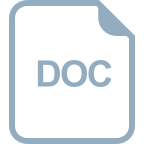
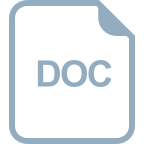
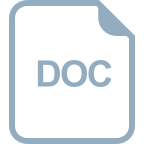










