linux 有P1,P2,P3三个进程,P1和P2负责从键盘接收字符串,均发送给P3,P3接收到字符串,根据发送方分别显示”P3 received *** from P1(或P2)" ;分别用管道通信,消息队列和共享存储三种通信方式实现。形成完整的可运行代码,不需要注释。
时间: 2023-12-06 21:03:10 浏览: 80
使用管道通信方式实现:
P1:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd[2];
char buf[100];
if(pipe(fd) == -1) {
perror("pipe");
exit(1);
}
while(1) {
printf("Enter message for P3 (from P1):\n");
scanf("%s", buf);
write(fd[1], buf, strlen(buf)+1);
}
return 0;
}
```
P2:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd[2];
char buf[100];
if(pipe(fd) == -1) {
perror("pipe");
exit(1);
}
while(1) {
printf("Enter message for P3 (from P2):\n");
scanf("%s", buf);
write(fd[1], buf, strlen(buf)+1);
}
return 0;
}
```
P3:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd1[2], fd2[2];
char buf[100];
if(pipe(fd1) == -1) {
perror("pipe1");
exit(1);
}
if(pipe(fd2) == -1) {
perror("pipe2");
exit(1);
}
while(1) {
read(fd1[0], buf, sizeof(buf));
printf("P3 received %s from P1\n", buf);
read(fd2[0], buf, sizeof(buf));
printf("P3 received %s from P2\n", buf);
}
return 0;
}
```
使用消息队列通信方式实现:
P1:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/msg.h>
struct msgbuf {
long mtype;
char mtext[100];
};
int main()
{
key_t key;
int msgid;
struct msgbuf buf;
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT|0666);
while(1) {
printf("Enter message for P3 (from P1):\n");
scanf("%s", buf.mtext);
buf.mtype = 1;
msgsnd(msgid, &buf, strlen(buf.mtext)+1, 0);
}
return 0;
}
```
P2:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/msg.h>
struct msgbuf {
long mtype;
char mtext[100];
};
int main()
{
key_t key;
int msgid;
struct msgbuf buf;
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT|0666);
while(1) {
printf("Enter message for P3 (from P2):\n");
scanf("%s", buf.mtext);
buf.mtype = 2;
msgsnd(msgid, &buf, strlen(buf.mtext)+1, 0);
}
return 0;
}
```
P3:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/msg.h>
struct msgbuf {
long mtype;
char mtext[100];
};
int main()
{
key_t key;
int msgid;
struct msgbuf buf;
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT|0666);
while(1) {
msgrcv(msgid, &buf, sizeof(buf), 1, 0);
printf("P3 received %s from P1\n", buf.mtext);
msgrcv(msgid, &buf, sizeof(buf), 2, 0);
printf("P3 received %s from P2\n", buf.mtext);
}
return 0;
}
```
使用共享存储通信方式实现:
P1:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
struct shared_use_st {
int written;
char text[100];
};
int main()
{
int running = 1;
void *shm = NULL;
struct shared_use_st *shared;
char buffer[BUFSIZ];
int shmid;
shmid = shmget((key_t)1234, sizeof(struct shared_use_st), 0666|IPC_CREAT);
if(shmid == -1) {
fprintf(stderr, "shmget failed\n");
exit(EXIT_FAILURE);
}
shm = shmat(shmid, (void *)0, 0);
if(shm == (void *)-1) {
fprintf(stderr, "shmat failed\n");
exit(EXIT_FAILURE);
}
shared = (struct shared_use_st *)shm;
shared->written = 0;
while(running) {
printf("Enter message for P3 (from P1):\n");
scanf("%s", buffer);
while(shared->written == 1) {
sleep(1);
printf("Waiting for P3 to read...\n");
}
strncpy(shared->text, buffer, 100);
shared->written = 1;
}
if(shmdt(shm) == -1) {
fprintf(stderr, "shmdt failed\n");
exit(EXIT_FAILURE);
}
if(shmctl(shmid, IPC_RMID, 0) == -1) {
fprintf(stderr, "shmctl(IPC_RMID) failed\n");
exit(EXIT_FAILURE);
}
return 0;
}
```
P2:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
struct shared_use_st {
int written;
char text[100];
};
int main()
{
int running = 1;
void *shm = NULL;
struct shared_use_st *shared;
char buffer[BUFSIZ];
int shmid;
shmid = shmget((key_t)1234, sizeof(struct shared_use_st), 0666|IPC_CREAT);
if(shmid == -1) {
fprintf(stderr, "shmget failed\n");
exit(EXIT_FAILURE);
}
shm = shmat(shmid, (void *)0, 0);
if(shm == (void *)-1) {
fprintf(stderr, "shmat failed\n");
exit(EXIT_FAILURE);
}
shared = (struct shared_use_st *)shm;
shared->written = 0;
while(running) {
printf("Enter message for P3 (from P2):\n");
scanf("%s", buffer);
while(shared->written == 1) {
sleep(1);
printf("Waiting for P3 to read...\n");
}
strncpy(shared->text, buffer, 100);
shared->written = 1;
}
if(shmdt(shm) == -1) {
fprintf(stderr, "shmdt failed\n");
exit(EXIT_FAILURE);
}
if(shmctl(shmid, IPC_RMID, 0) == -1) {
fprintf(stderr, "shmctl(IPC_RMID) failed\n");
exit(EXIT_FAILURE);
}
return 0;
}
```
P3:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
struct shared_use_st {
int written;
char text[100];
};
int main()
{
int running = 1;
void *shm = NULL;
struct shared_use_st *shared;
int shmid;
shmid = shmget((key_t)1234, sizeof(struct shared_use_st), 0666|IPC_CREAT);
if(shmid == -1) {
fprintf(stderr, "shmget failed\n");
exit(EXIT_FAILURE);
}
shm = shmat(shmid, 0, 0);
if(shm == (void *)-1) {
fprintf(stderr, "shmat failed\n");
exit(EXIT_FAILURE);
}
shared = (struct shared_use_st *)shm;
while(running) {
if(shared->written == 1) {
printf("P3 received %s from P1\n", shared->text);
shared->written = 0;
}
if(shared->written == 2) {
printf("P3 received %s from P2\n", shared->text);
shared->written = 0;
}
sleep(1);
}
if(shmdt(shm) == -1) {
fprintf(stderr, "shmdt failed\n");
exit(EXIT_FAILURE);
}
if(shmctl(shmid, IPC_RMID, 0) == -1) {
fprintf(stderr, "shmctl(IPC_RMID) failed\n");
exit(EXIT_FAILURE);
}
return 0;
}
```
阅读全文
相关推荐
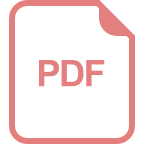












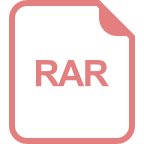
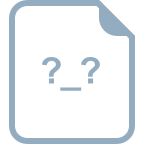