三维装箱代码
时间: 2023-07-16 16:14:34 浏览: 95
三维装箱问题是一个NP难问题,没有一种通用的高效算法可以解决。但是可以使用启发式算法或近似算法来解决。
以下是一个简单的启发式算法的伪代码:
1. 初始化一个空的容器列表
2. 将所有物品按照体积从大到小排序
3. 对于每个物品:
a. 遍历所有容器,找到能够放下该物品的最小容器
b. 如果找到了,将该物品放入该容器中
c. 如果没有找到,创建一个新的容器,并将该物品放入其中
4. 输出容器列表
具体实现可以参考以下的Python代码实现:
```python
class Box:
def __init__(self, width, height, depth):
self.width = width
self.height = height
self.depth = depth
self.items = []
def add_item(self, item):
self.items.append(item)
def get_volume(self):
return self.width * self.height * self.depth
class Item:
def __init__(self, id, width, height, depth):
self.id = id
self.width = width
self.height = height
self.depth = depth
self.volume = width * height * depth
def pack_items(items):
boxes = []
items.sort(key=lambda x: x.volume, reverse=True)
for item in items:
fit_box = None
for box in boxes:
if box.width >= item.width and box.height >= item.height and box.depth >= item.depth:
if fit_box is None or box.get_volume() < fit_box.get_volume():
fit_box = box
if fit_box is None:
fit_box = Box(item.width, item.height, item.depth)
boxes.append(fit_box)
fit_box.add_item(item)
return boxes
# Example usage
items = [
Item(1, 20, 30, 40),
Item(2, 15, 25, 35),
Item(3, 10, 20, 30),
Item(4, 5, 10, 15)
]
boxes = pack_items(items)
for i, box in enumerate(boxes):
print(f"Box {i+1}:")
for item in box.items:
print(f" Item {item.id}: ({item.width}, {item.height}, {item.depth})")
```
这段代码使用了一个简单的贪心算法,将物品按照体积从大到小排序,然后将每个物品放入能够放得下该物品的最小容器中。这种方法在一些情况下可能会产生不理想的结果,但是在许多实际应用中已经被证明是有效的。
阅读全文
相关推荐
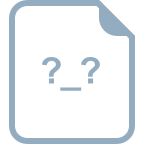
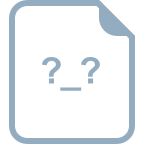
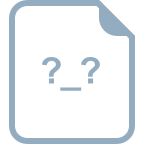







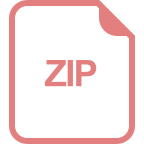
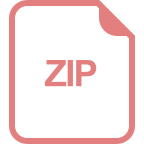

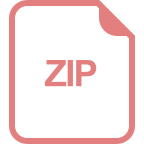
