提供一个完整的使用启发式算法的三维装箱代码
时间: 2023-06-19 11:09:51 浏览: 44
以下是一个使用启发式算法(First Fit Decreasing)的三维装箱代码的简单实现,仅供参考:
```python
class Box:
def __init__(self, width, height, depth):
self.width = width
self.height = height
self.depth = depth
class Item:
def __init__(self, index, width, height, depth):
self.index = index
self.width = width
self.height = height
self.depth = depth
class PackedItem:
def __init__(self, item, x, y, z):
self.item = item
self.x = x
self.y = y
self.z = z
class Bin:
def __init__(self, width, height, depth):
self.width = width
self.height = height
self.depth = depth
self.items = []
def pack_items(self, items):
items.sort(key=lambda item: max(item.width, item.height, item.depth), reverse=True)
for item in items:
if not self.pack_item(item):
return False
return True
def pack_item(self, item):
for x in range(self.width - item.width + 1):
for y in range(self.height - item.height + 1):
for z in range(self.depth - item.depth + 1):
if self.can_pack_item(item, x, y, z):
self.items.append(PackedItem(item, x, y, z))
self.mark_as_packed(item, x, y, z)
return True
return False
def can_pack_item(self, item, x, y, z):
for packed_item in self.items:
if x + item.width <= packed_item.x or packed_item.x + packed_item.item.width <= x:
continue
if y + item.height <= packed_item.y or packed_item.y + packed_item.item.height <= y:
continue
if z + item.depth <= packed_item.z or packed_item.z + packed_item.item.depth <= z:
continue
return False
return True
def mark_as_packed(self, item, x, y, z):
pass # 可以在这里添加代码,标记物品已经被打包
def pack_items(items, bin_width, bin_height, bin_depth):
bin = Bin(bin_width, bin_height, bin_depth)
if not bin.pack_items(items):
raise Exception("无法将所有物品打包到箱子中")
return bin.items
# 示例用法
items = [
Item(1, 5, 3, 4),
Item(2, 2, 2, 2),
Item(3, 4, 6, 2),
Item(4, 3, 5, 3),
Item(5, 5, 3, 1),
Item(6, 2, 4, 3),
Item(7, 4, 4, 4),
Item(8, 1, 1, 1)
]
packed_items = pack_items(items, 10, 10, 10)
for packed_item in packed_items:
print(f"物品{packed_item.item.index}被打包在({packed_item.x}, {packed_item.y}, {packed_item.z})")
```
该代码使用了一个简单的三维“箱子-物品”模型来表示问题。Box类表示一个箱子,Item类表示一个待打包的物品,PackedItem类表示已经被打包的物品,Bin类表示一个箱子中已经被打包的物品集合。
该代码实现了First Fit Decreasing启发式算法,即先按照物品的最大尺寸排序,然后按顺序尝试将每一个物品打包到箱子中。如果打包成功,则将该物品添加到Bin.items列表中,并标记该物品已经被打包;如果打包失败,则返回False表示无法将所有物品打包到箱子中。
相关推荐










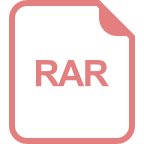