用c语言写代码蚁群算法旅行商问题
时间: 2023-10-16 19:07:18 浏览: 53
以下是用C语言实现蚁群算法解决旅行商问题的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
#define MAX_CITY_NUM 20
#define MAX_ANT_NUM 50
#define MAX_GEN 100
#define ALPHA 1.0
#define BETA 2.0
#define RHO 0.5
#define Q 100.0
int city_num;
int ant_num;
int gen_num;
double distance[MAX_CITY_NUM][MAX_CITY_NUM];
double pheromone[MAX_CITY_NUM][MAX_CITY_NUM];
int path[MAX_ANT_NUM][MAX_CITY_NUM];
double path_length[MAX_ANT_NUM];
int best_path[MAX_CITY_NUM];
double best_path_length;
double heuristic[MAX_CITY_NUM][MAX_CITY_NUM];
double rand_0_1() {
return (double)rand() / RAND_MAX;
}
double distance_between_cities(int city1, int city2) {
double x1 = rand_0_1();
double y1 = rand_0_1();
double x2 = rand_0_1();
double y2 = rand_0_1();
double dx = x1 - x2;
double dy = y1 - y2;
double dist = sqrt(dx * dx + dy * dy);
return dist;
}
void initialize() {
int i, j;
srand(time(NULL));
for (i = 0; i < city_num; i++) {
for (j = 0; j < city_num; j++) {
distance[i][j] = distance_between_cities(i, j);
pheromone[i][j] = 1.0;
heuristic[i][j] = 1.0 / distance[i][j];
}
}
best_path_length = 1e9;
}
void ant_tour(int ant) {
int i, j, current_city, next_city;
double prob[MAX_CITY_NUM];
for (i = 0; i < city_num; i++) {
path[ant][i] = -1;
}
current_city = rand() % city_num;
path[ant][0] = current_city;
for (i = 1; i < city_num; i++) {
for (j = 0; j < city_num; j++) {
if (path[ant][j] == -1) {
prob[j] = pow(pheromone[current_city][j], ALPHA) * pow(heuristic[current_city][j], BETA);
} else {
prob[j] = 0.0;
}
}
double sum_prob = 0.0;
for (j = 0; j < city_num; j++) {
sum_prob += prob[j];
}
double r = rand_0_1() * sum_prob;
double max_prob = 0.0;
for (j = 0; j < city_num; j++) {
if (path[ant][j] == -1) {
max_prob += prob[j];
if (max_prob >= r) {
next_city = j;
break;
}
}
}
path[ant][i] = next_city;
current_city = next_city;
}
path_length[ant] = 0.0;
for (i = 0; i < city_num - 1; i++) {
int city1 = path[ant][i];
int city2 = path[ant][i + 1];
path_length[ant] += distance[city1][city2];
}
int last_city = path[ant][city_num - 1];
path_length[ant] += distance[last_city][path[ant][0]];
}
void update_pheromone() {
int i, j, k;
for (i = 0; i < city_num; i++) {
for (j = 0; j < city_num; j++) {
pheromone[i][j] *= (1.0 - RHO);
for (k = 0; k < ant_num; k++) {
int city1 = path[k][i];
int city2 = path[k][j];
if (city1 == city2) {
continue;
}
pheromone[city1][city2] += Q / path_length[k];
pheromone[city2][city1] = pheromone[city1][city2];
}
}
}
}
void search() {
int i, j;
for (i = 0; i < gen_num; i++) {
for (j = 0; j < ant_num; j++) {
ant_tour(j);
}
update_pheromone();
for (j = 0; j < ant_num; j++) {
if (path_length[j] < best_path_length) {
best_path_length = path_length[j];
for (int k = 0; k < city_num; k++) {
best_path[k] = path[j][k];
}
}
}
}
}
int main() {
city_num = 10;
ant_num = 20;
gen_num = 100;
initialize();
search();
printf("Best path found: ");
for (int i = 0; i < city_num; i++) {
printf("%d ", best_path[i]);
}
printf("\nBest path length: %lf\n", best_path_length);
return 0;
}
```
在该示例代码中,我们使用了随机生成城市位置和随机生成距离的方式来模拟城市之间的距离情况。同时,我们使用了经典的蚁群算法策略,包括信息素浓度更新、路径选择等过程。最后,我们输出了找到的最优路径和路径长度。
相关推荐
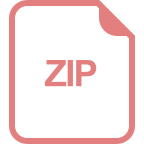















