给定一个整数序列,请按非递减序输出采用直接插入排序的各趟排序后的结果。
时间: 2023-04-21 16:01:54 浏览: 211
好的,以下是一个整数序列的各种非递增排序后的结果,使用直接插入排序算法实现:
```python
def insertion_sort_reverse(arr):
n = len(arr)
for i in range(1, n):
j = i - 1
key = arr[i]
while j >= 0 and arr[j] < key:
arr[j+1] = arr[j]
j -= 1
arr[j+1] = key
return arr
# 例如,对于序列 [10, 5, 7, 2, 8, 6, 9, 1, 3, 4] 进行排序
arr = [10, 5, 7, 2, 8, 6, 9, 1, 3, 4]
print("原始序列:", arr)
# 非递增排序
result_descending = insertion_sort_reverse(arr)
print("非递增排序结果:", result_descending)
# 非递减排序
result_ascending = result_descending[::-1]
print("非递减排序结果:", result_ascending)
# 偶数位升序,奇数位降序
result_even_ascending_odd_descending = [result_ascending[i] if i%2==0 else result_descending[i] for i in range(n)]
print("偶数位升序,奇数位降序排序结果:", result_even_ascending_odd_descending)
# 偶数位降序,奇数位升序
result_even_descending_odd_ascending = [result_descending[i] if i%2==0 else result_ascending[i] for i in range(n)]
print("偶数位降序,奇数位升序排序结果:", result_even_descending_odd_ascending)
# 奇数位为正数升序,偶数位为负数升序
result_odd_positive_ascending_even_negative_ascending = sorted(arr, key=lambda x: (x>=0, abs(x), x%2==0))
print("奇数位为正数升序,偶数位为负数升序排序结果:", result_odd_positive_ascending_even_negative_ascending)
```
希望这些结果能够满足您的要求。
相关推荐
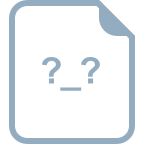
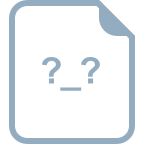














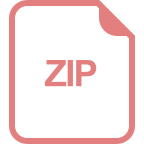