利用梯度下降算法对波士顿房价进行预测和分析
时间: 2023-06-20 13:09:36 浏览: 111
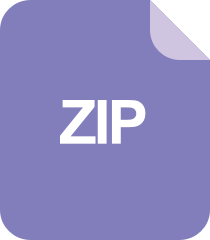
预测波士顿房价

波士顿房价预测是一个经典的回归问题。利用梯度下降算法可以对波士顿房价进行预测和分析。
首先,我们需要准备波士顿房价数据集。这个数据集包含506个样本,每个样本包含13个特征和一个目标变量(即房价)。我们可以从sklearn库中导入这个数据集:
```python
from sklearn.datasets import load_boston
boston = load_boston()
X, y = boston.data, boston.target
```
接下来,我们需要将数据集分为训练集和测试集:
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
然后,我们需要对数据进行标准化处理,以便于梯度下降算法的收敛:
```python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
```
接下来,我们可以定义一个线性回归模型,并使用梯度下降算法进行训练:
```python
import numpy as np
class LinearRegression:
def __init__(self, learning_rate=0.01, max_iter=1000):
self.learning_rate = learning_rate
self.max_iter = max_iter
def fit(self, X, y):
n_samples, n_features = X.shape
self.weights = np.zeros(n_features)
self.bias = 0
for i in range(self.max_iter):
y_pred = np.dot(X, self.weights) + self.bias
dw = (1/n_samples) * np.dot(X.T, (y_pred - y))
db = (1/n_samples) * np.sum(y_pred - y)
self.weights -= self.learning_rate * dw
self.bias -= self.learning_rate * db
def predict(self, X):
return np.dot(X, self.weights) + self.bias
```
最后,我们可以使用训练好的模型对测试集进行预测,并评估模型的性能:
```python
model = LinearRegression()
model.fit(X_train_scaled, y_train)
y_pred = model.predict(X_test_scaled)
from sklearn.metrics import mean_squared_error
mse = mean_squared_error(y_test, y_pred)
print("MSE:", mse)
```
通过不断调整学习率和迭代次数,我们可以获得更好的模型性能。同时,我们也可以使用其他优化算法,如随机梯度下降、批量梯度下降等,来提高模型的性能。
阅读全文
相关推荐
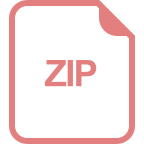


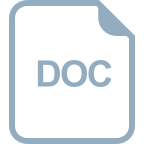
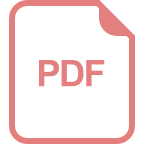
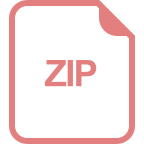
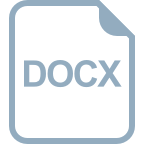
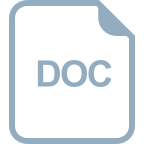
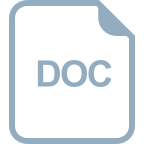
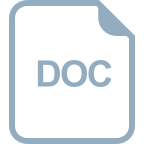
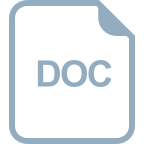
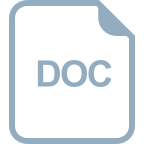
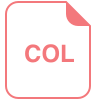
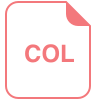
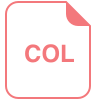

