从文件中读取两个数存入两个链表
时间: 2023-07-04 14:19:09 浏览: 134
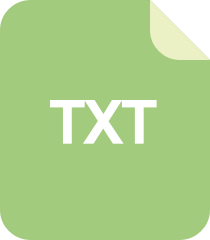
读文件到链表
好的,我可以帮你解决这个问题。
假设你有一个文件名为input.txt,里面包含两个数,每个数占一行,可以按照以下方法读取这两个数,并将它们存入两个链表中:
```python
# 定义链表节点类
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 读取文件,并将数字存入链表中
def read_nums_from_file(filename):
# 定义两个链表头节点
head1, head2 = ListNode(), ListNode()
cur1, cur2 = head1, head2
with open(filename, 'r') as f:
lines = f.readlines()
# 读取第一个数,并存入链表1中
cur1.val = int(lines[0].strip())
for i in range(1, len(lines)):
# 读取第二个数,并存入链表2中
cur2.val = int(lines[i].strip())
# 如果还有剩余数字,继续创建新节点存入链表2中
if i != len(lines) - 1:
cur2.next = ListNode()
cur2 = cur2.next
# 如果链表1中还有剩余数字,继续创建新节点存入链表1中
if i != 1:
cur1.next = ListNode()
cur1 = cur1.next
return head1, head2
```
这个函数将返回两个链表的头节点。你可以使用这些节点来访问每个链表中的数字。
阅读全文
相关推荐
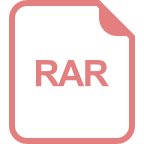




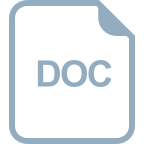
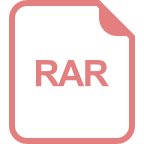
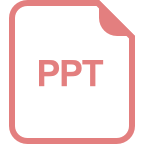
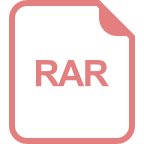
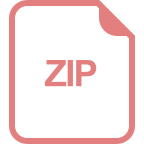
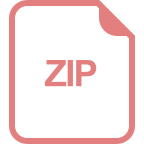
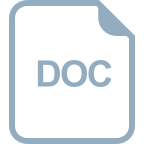




