在linux中,两个应用之间如何使用串口进行通讯
时间: 2024-09-13 08:05:04 浏览: 34
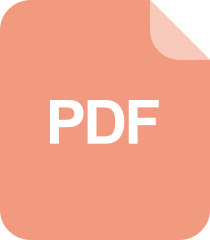
串口通讯在Linux操作系统中的应用.pdf
在Linux中,两个应用之间使用串口进行通信,通常需要通过串口设备文件来实现。以下是使用串口进行通信的基本步骤:
1. 确认串口设备:首先,你需要确认你的串口设备文件名,通常为`/dev/ttyS0`, `/dev/ttyUSB0`, `/dev/ttyACM0`等。这可以通过`dmesg`命令来查看,或者使用`ls /dev/tty*`列出所有串口设备。
2. 打开串口设备文件:应用程序需要以读写模式打开串口设备文件。例如,在C语言中,可以使用`open()`函数来打开设备文件。
3. 配置串口:使用`ioctl()`函数来配置串口的各种参数,如波特率、数据位、停止位、校验位等。
4. 读写数据:配置完串口后,就可以使用`read()`和`write()`函数来读取和发送数据了。
5. 关闭串口:通信结束后,需要使用`close()`函数关闭串口设备文件。
示例代码(C语言):
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open_port: Unable to open /dev/ttyS0 - ");
return(-1);
}
struct termios options;
tcgetattr(fd, &options);
// 设置波特率
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
// 设置数据位数
options.c_cflag &= ~CSIZE; // Mask the character size bits
options.c_cflag |= CS8;
// 设置为无奇偶校验位
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
// 设置为一个字符一个停止位
options.c_cflag &= ~CRTSCTS;
// 应用设置
tcsetattr(fd, TCSANOW, &options);
// 写数据
char *write_buffer = "Hello World";
write(fd, write_buffer, sizeof(write_buffer));
// 读数据
char read_buffer[100];
read(fd, read_buffer, sizeof(read_buffer));
printf("Received: %s\n", read_buffer);
close(fd);
return 0;
}
```
阅读全文
相关推荐
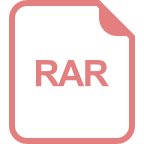
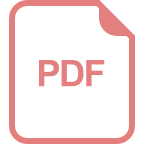
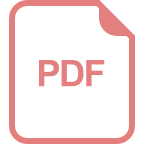
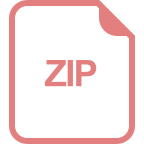
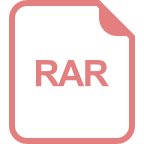
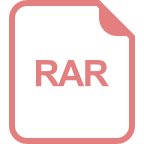
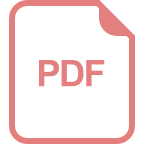
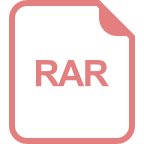
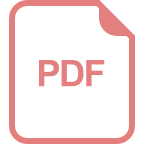
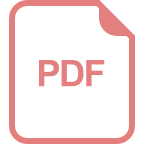
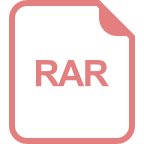
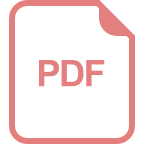
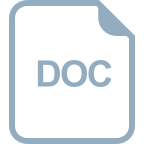
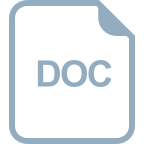
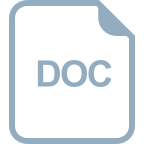
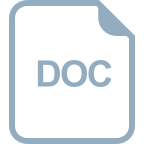
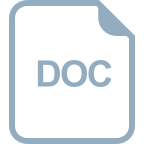
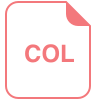