unity webgl 和vue通信
时间: 2023-09-01 11:11:07 浏览: 81
Unity WebGL 和 Vue 可以通过以下方式进行通信:
1. 使用 postMessage() 方法
在 Unity WebGL 中,可以使用 Unity 的 SendMessage() 方法将消息发送到 Vue 应用程序中。在 Vue 应用程序中,可以使用 window.addEventListener() 监听消息事件,然后根据消息内容进行相应的操作。
Unity 中的代码示例:
```
function sendMessageToVue(message) {
var iframe = window.parent.document.getElementById('your-iframe-id');
iframe.contentWindow.postMessage(message, '*');
}
```
在 Vue 应用程序中的代码示例:
```
mounted() {
window.addEventListener('message', this.handleUnityMessage);
},
methods: {
handleUnityMessage(event) {
if (event.origin !== 'https://your-unity-webgl-domain.com') {
return;
}
const message = event.data;
// 根据消息内容进行相应的操作
}
}
```
2. 使用 Vue.js Unity Web Player 插件
Vue.js Unity Web Player 插件提供了一种更方便的方式来在 Vue 应用程序中嵌入 Unity WebGL 游戏,并与之进行通信。该插件可以在 Vue 应用程序中直接使用 Unity API。
首先,需要安装 vue-unity-webgl 插件:
```
npm install vue-unity-webgl --save
```
然后,在 Vue 应用程序中使用该插件:
```
<template>
<div>
<unity-webgl :game-width="800" :game-height="600"
src="https://your-unity-webgl-domain.com/your-game.json"
@unityMessage="handleUnityMessage"></unity-webgl>
</div>
</template>
<script>
import UnityWebgl from 'vue-unity-webgl';
export default {
components: {
UnityWebgl,
},
methods: {
handleUnityMessage(message) {
// 根据消息内容进行相应的操作
},
},
};
</script>
```
在 Unity 中,需要使用 Unity 的 SendMessage() 方法来发送消息到 Vue 应用程序中:
```
function sendMessageToVue(message) {
unityInstance.SendMessage('VueProxy', 'ReceiveMessage', message);
}
```
在 Vue 应用程序中,需要在组件中定义一个名为 ReceiveMessage 的方法来接收 Unity 发送的消息。插件会自动将接收到的消息作为参数传入该方法中:
```
<template>
<div></div>
</template>
<script>
export default {
methods: {
ReceiveMessage(message) {
// 根据消息内容进行相应的操作
},
},
};
</script>
```
相关推荐
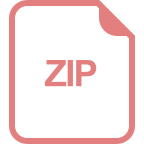













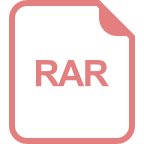