var强转Dictionary<string, int>
时间: 2023-12-19 21:07:24 浏览: 93
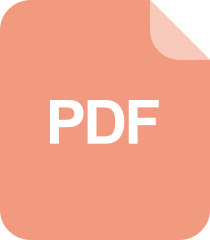
C#中Dictionary几种遍历的实现代码
在C#中,可以使用强制类型转换将一个变量从一种类型转换为另一种类型。要将一个 var 类型的变量强制转换为 Dictionary<string, int> 类型,可以使用以下代码:
```
var myVar = new Dictionary<string, int>();
// 添加一些键值对到 myVar ...
Dictionary<string, int> myDict = (Dictionary<string, int>)myVar;
```
在上面的代码中,我们首先创建一个 var 类型的变量 myVar,然后将其添加一些键值对。然后我们使用强制类型转换将 myVar 转换为 Dictionary<string, int> 类型,并将结果存储在 myDict 变量中。请注意,如果 myVar 变量实际上不是 Dictionary<string, int> 类型,那么这个转换将会失败并抛出一个异常。
阅读全文
相关推荐
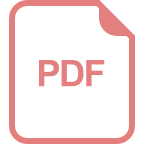
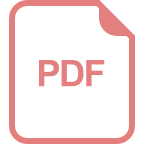





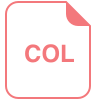
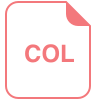




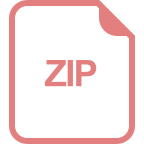
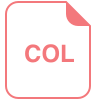


