设计一处程序要求从系统文件“/etc/passwd”读取偏移量从100至200之间的字符,写入“/tmp/pass”文件。
时间: 2023-11-27 17:02:46 浏览: 48
以下是一个简单的Python程序实现:
```python
with open('/etc/passwd', 'r') as file:
content = file.read()[100:201]
with open('/tmp/pass', 'w') as file:
file.write(content)
```
该程序首先打开文件“/etc/passwd”,读取其内容,并将偏移量在100到200之间的字符存储在变量“content”中。然后,它打开文件“/tmp/pass”,并将“content”变量的内容写入该文件中。
相关问题
用Linux设计一处程序要求从系统文件“/etc/passwd”读取偏移量从100至200之间的字符,写入“/tmp/pass”文件。
可以Linux中的dd命令来实现这个需求。dd命令可以从一个文件中读取指定的字节范围,并将其写入到另一个文件中。
下面是实现的步骤:
1. 打开终端,进入命令行界面。
2. 输入以下命令,将“/etc/passwd”文件中偏移量为100至200之间的字符写入“/tmp/pass”文件中:
```
dd if=/etc/passwd of=/tmp/pass bs=1 skip=100 count=100
```
解释一下这个命令:
- `if=/etc/passwd`:表示输入文件为“/etc/passwd”。
- `of=/tmp/pass`:表示输出文件为“/tmp/pass”。
- `bs=1`:表示每次读写的块大小为1字节。
- `skip=100`:表示跳过输入文件前100个字节。
- `count=100`:表示从输入文件中读取100个字节。
执行完上述命令后,就可以在“/tmp/pass”文件中查看偏移量为100至200之间的字符了。
用LinuxC语言设计一处程序要求从系统文件“/etc/passwd”读取偏移量从100至200之间的字符,写入“/tmp/pass”文件。
以下是一个简单的C程序,实现了你想要的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#define BUF_SIZE 1024
int main() {
int fd1, fd2;
char buf[BUF_SIZE];
ssize_t nread;
// 打开 /etc/passwd 文件
if ((fd1 = open("/etc/passwd", O_RDONLY)) < 0) {
perror("open /etc/passwd failed");
exit(1);
}
// 创建 /tmp/pass 文件
if ((fd2 = open("/tmp/pass", O_WRONLY | O_CREAT | O_TRUNC, 0644)) < 0) {
perror("create /tmp/pass failed");
exit(1);
}
// 读取文件偏移量在 100 至 200 之间的字符,并写入 /tmp/pass 文件
lseek(fd1, 100, SEEK_SET);
while ((nread = read(fd1, buf, BUF_SIZE)) > 0) {
if (lseek(fd1, 100, SEEK_CUR) == -1) {
perror("lseek failed");
exit(1);
}
if (write(fd2, buf, nread) != nread) {
perror("write failed");
exit(1);
}
if (lseek(fd1, 100, SEEK_CUR) == -1) {
perror("lseek failed");
exit(1);
}
}
if (nread == -1) {
perror("read failed");
exit(1);
}
// 关闭文件
close(fd1);
close(fd2);
return 0;
}
```
这个程序首先打开了 /etc/passwd 文件和 /tmp/pass 文件,然后使用 lseek 函数将文件指针移到偏移量为 100 的位置开始读取,并将读到的字符写入 /tmp/pass 文件中。最后关闭了两个文件。注意,这里使用了一些错误处理来确保程序的正确性。
阅读全文
相关推荐
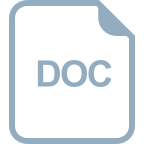
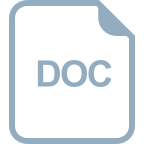
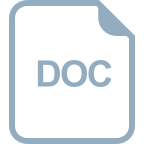

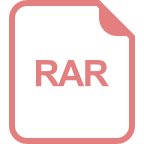
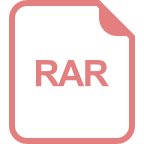
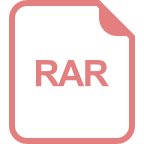
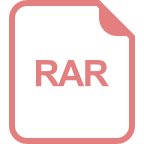
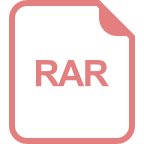
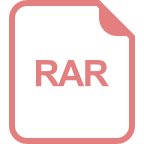
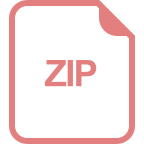
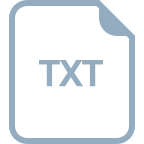
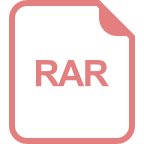
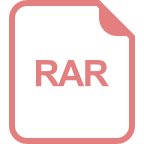
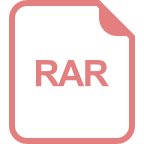
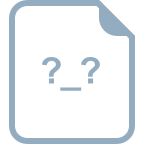
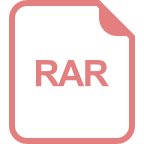