@org.junit.Test public void test08() { // 所有的 List<PeiJianWeiHu> peiJianWeiHuList = carRemarksDao.findPeiJianWeiHu(); // 授权的 List<CarAccessories> carAccessoriesList = carRemarksDao.selectByProTypeAndFirLevSort("车身贴", "国潮贴"); // 保存的 List<PeiJianWeiHu> newList = new ArrayList<>(); for (PeiJianWeiHu peiJianWeiHu : peiJianWeiHuList) { for (CarAccessories carAccessories : carAccessoriesList) { if (carAccessories.getClassify().equals(peiJianWeiHu.getFenLei()) && carAccessories.getDisplayName().equals(peiJianWeiHu.getXianShiMingCheng())) { newList.add(peiJianWeiHu); } } } System.out.println("newList = " + newList); }
时间: 2024-04-19 12:23:48 浏览: 6
这段代码的作用是从数据库中获取相关数据并进行筛选,最后将符合条件的数据保存到新的列表`newList`中,并打印出来。具体的代码逻辑如下:
1. 通过调用`carRemarksDao.findPeiJianWeiHu()`方法,获取所有的`PeiJianWeiHu`对象列表,保存到`peiJianWeiHuList`中。
2. 通过调用`carRemarksDao.selectByProTypeAndFirLevSort("车身贴", "国潮贴")`方法,获取符合条件的`CarAccessories`对象列表,保存到`carAccessoriesList`中。
3. 创建一个新的空列表`newList`,用于保存符合条件的`PeiJianWeiHu`对象。
4. 遍历`peiJianWeiHuList`和`carAccessoriesList`,对比每个`PeiJianWeiHu`对象和每个`CarAccessories`对象的属性,如果满足条件,则将该`PeiJianWeiHu`对象添加到`newList`中。
5. 打印出`newList`的内容。
请注意,这段代码是一个JUnit测试方法,它通常用于测试某个类或者方法的功能是否正确。
相关问题
</dependency> <dependency> <groupId>junit</groupId> <dependency> <groupId>org.powermock</groupId> <artifactId>powermock-module-junit4</artifactId> <version>${powermock.version}</version> <scope>test</scope> </dependency>.测试覆盖率达到100% <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency>
好的,那么我们可以先针对 `TaskPollRunnable` 类中的 `pollTasks` 方法写一个单元测试。由于该方法的返回值是通过调用 `ftpManagerClient.getCleanTasks(num)` 来获取的,所以我们需要使用 mock 对象来模拟这个调用。
首先,我们需要在 `pom.xml` 中引入 JUnit 和 PowerMock 的依赖:
```xml
<dependencies>
<!-- JUnit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<!-- PowerMock -->
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-module-junit4</artifactId>
<version>${powermock.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-api-mockito2</artifactId>
<version>${powermock.version}</version>
<scope>test</scope>
</dependency>
</dependencies>
```
然后,我们可以编写如下的测试代码:
```java
@RunWith(PowerMockRunner.class)
@PrepareForTest({FtpManagerClient.class})
public class TaskPollRunnableTest {
private CleanTaskPollService cleanTaskPollService;
private FtpManagerClient ftpManagerClient;
@Before
public void setUp() throws Exception {
cleanTaskPollService = new CleanTaskPollService(/* 传入参数 */);
ftpManagerClient = mock(FtpManagerClient.class);
Whitebox.setInternalState(cleanTaskPollService, "ftpManagerClient", ftpManagerClient);
}
@Test
public void testPollTasks() throws Exception {
// 构造 mock 返回值
FileCleanTaskResponse response = new FileCleanTaskResponse();
List<FileCleanTask> tasks = new ArrayList<>();
FileCleanTask task1 = new FileCleanTask();
task1.setCleanDirs(Arrays.asList("/path/to/dir1", "/path/to/dir2"));
tasks.add(task1);
response.setCleanTasks(tasks);
// 设置 mock 对象的行为
Response<FileCleanTaskResponse> mockResponse = Response.success(response);
Call<FileCleanTaskResponse> mockCall = mock(Call.class);
when(ftpManagerClient.getCleanTasks(anyInt())).thenReturn(mockCall);
when(mockCall.execute()).thenReturn(mockResponse);
// 调用被测试方法
FileCleanTaskResponse result = cleanTaskPollService.pollTasks(1);
// 验证结果
assertEquals(response, result);
}
}
```
这个测试用例使用了 PowerMock 框架来 mock `FtpManagerClient` 类的实例,并设置其调用 `getCleanTasks` 方法时返回指定的 mock 对象。因此,我们可以在测试中模拟出 `getCleanTasks` 方法的返回值,来验证 `pollTasks` 方法的正确性。
当然,这只是针对一个小小的方法的测试,你还需要根据代码的实际情况编写更多的测试用例,以确保代码的正确性。
修改代码中的错误package com.zxq; import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; import com.zxq.domain.User; import org.junit.jupiter.api.Test; import org.springframework.boot.test.context.SpringBootTest; import java.util.HashMap; import java.util.List; import java.util.Map; @SpringBootTest class SpringbootMybatisplusZbApplicationTests { @Test void contextLoads() { } public void selectByName(){ Map<String,Object> columnMap=new HashMap<>(); columnMap.put("name","admin"); List<User> list=userMapper.selectByMap(columnMap) } @Test public void selectByProperty(){ QueryWrapper<User> queryWrapper=new QueryWrapper<>(); // queryWrapper.like("name","a"); queryWrapper.select("name","sex");//投影查询 List<User> list=uerMapper.selectList(queryWrapper); System.out.println(list); } }
在代码中,缺少了一个分号,导致出现了语法错误。在selectByName()方法中,将最后一行代码中的分号添加上即可,修改后的代码如下:
```
package com.zxq;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.zxq.domain.User;
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@SpringBootTest
class SpringbootMybatisplusZbApplicationTests {
@Test
void contextLoads() {
}
public void selectByName(){
Map<String,Object> columnMap=new HashMap<>();
columnMap.put("name","admin");
List<User> list=userMapper.selectByMap(columnMap);
}
@Test
public void selectByProperty(){
QueryWrapper<User> queryWrapper=new QueryWrapper<>();
// queryWrapper.like("name","a");
queryWrapper.select("name","sex");//投影查询
List<User> list=userMapper.selectList(queryWrapper);
System.out.println(list);
}
}
```
相关推荐
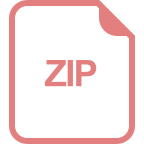













