用Java 定义一个二维空间的点类, 有横、纵坐标信息, 有计算两点之间距离的方法, 有将当前点的横、纵坐标移动一定距离到下一个位置的方法。 定义一个测试类测试定义的这两个方法。
时间: 2024-10-24 11:18:54 浏览: 32
在Java中,我们可以定义一个名为`Point`的二维空间点类,包含横坐标`x`和纵坐标`y`属性,以及两个辅助方法:`distanceTo`用于计算与其他点的距离,`move`用于移动到新的位置。这里是一个简单的实现:
```java
public class Point {
private double x;
private double y;
// 构造函数
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// 计算两点之间的欧氏距离
public double distanceTo(Point other) {
return Math.sqrt(Math.pow(this.x - other.x, 2) + Math.pow(this.y - other.y, 2));
}
// 移动到新的位置
public void move(double dx, double dy) {
this.x += dx;
this.y += dy;
}
// 获取坐标值以便于打印或查看
@Override
public String toString() {
return "Point (" + x + ", " + y + ")";
}
}
// 测试类TestPoint
public class TestPoint {
public static void main(String[] args) {
// 创建两个点
Point pointA = new Point(0, 0);
Point pointB = new Point(3, 4);
// 打印初始位置
System.out.println("Point A: " + pointA);
System.out.println("Point B: " + pointB);
// 计算并打印距离
double distance = pointA.distanceTo(pointB);
System.out.println("Distance between A and B: " + distance);
// 移动点A
pointA.move(2, 2);
// 移动点B
pointB.move(-2, -2);
// 再次打印位置
System.out.println("Moved points:");
System.out.println("Point A: " + pointA);
System.out.println("Point B: " + pointB);
}
}
```
在这个例子中,`TestPoint`类通过创建`Point`对象,并演示了如何使用它们的`distanceTo`方法计算距离,以及如何使用`move`方法改变点的位置。
阅读全文
相关推荐
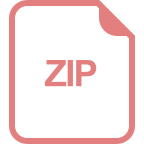
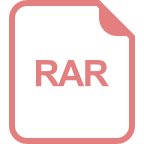
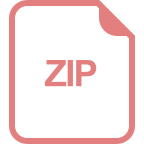









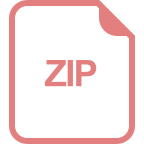

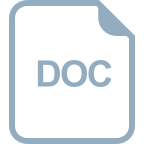
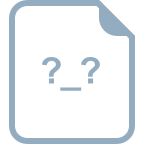
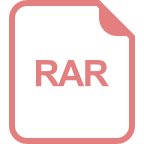
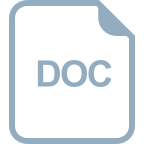