java定义二维空间的点类及线段,要求线段具有计算长度的功能,其他功能自拟,并测试。一步到位
时间: 2024-10-16 19:14:30 浏览: 36
在Java中,我们可以定义一个`Point`类表示二维空间中的点,以及一个`Segment`类表示连接两个点的线段。线段类会包含起始点和结束点,同时具备计算长度的方法。以下是简单的实现:
```java
import java.lang.Math;
// 定义二维空间的点类
class Point {
double x;
double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// 返回两点之间的距离,即线段长度
public double distanceTo(Point other) {
return Math.sqrt(Math.pow(this.x - other.x, 2) + Math.pow(this.y - other.y, 2));
}
}
// 定义线段类,包含开始和结束点
class Segment {
private Point start;
private Point end;
public Segment(Point start, Point end) {
this.start = start;
this.end = end;
}
// 获取线段长度
public double getLength() {
return start.distanceTo(end);
}
// 自定义功能,比如计算线段与x轴的交点
public Point intersectionWithXAxis() {
if (end.y == start.y) { // 平行于x轴
throw new IllegalArgumentException("Line is parallel to X-axis.");
} else {
double slope = (end.y - start.y) / (end.x - start.x); // 斜率
return new Point(0, start.y + slope * start.x); // 交点横坐标为0,纵坐标用斜率求得
}
}
}
public class Main {
public static void main(String[] args) {
// 创建并测试线段
Point pointA = new Point(0, 0);
Point pointB = new Point(3, 4);
Segment segment = new Segment(pointA, pointB);
System.out.println("Line segment length: " + segment.getLength());
try {
Point intersection = segment.intersectionWithXAxis();
System.out.println("Intersection with X-axis: (" + intersection.x + ", " + intersection.y + ")");
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
}
```
在这个例子中,我们创建了一个点类`Point`,用于存储二维坐标,并提供计算两点间距离的方法。`Segment`类包含了起点和终点,以及计算线段长度和查找与x轴交点的方法。
运行上述代码,你会看到线段的长度和与x轴交点的信息。请注意,这只是一个基础示例,你可以根据需要添加更多的功能,如计算角度、判断线段是否垂直等。
阅读全文
相关推荐














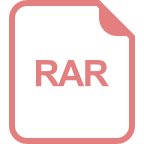

