.net framework 用多个声卡向多个音响同时播放不同文本语音
时间: 2023-08-16 11:05:11 浏览: 44
以下是一个使用NAudio库在C#中实现多个声卡向多个音响同时播放不同文本语音的示例:
```csharp
using System;
using System.Collections.Generic;
using System.Speech.Synthesis;
using NAudio.Wave;
namespace MultiSoundCardPlayer
{
class Program
{
static void Main(string[] args)
{
List<WaveOutEvent> soundCards = new List<WaveOutEvent>();
List<SpeechSynthesizer> speechSynthesizers = new List<SpeechSynthesizer>();
List<string> texts = new List<string>{"Hello", "world", "This", "is", "a", "test"};
// 打开多个声卡,创建多个实例
for (int i = 0; i < WaveOut.DeviceCount; i++)
{
WaveOutCapabilities capabilities = WaveOut.GetCapabilities(i);
Console.WriteLine($"Device {i}: {capabilities.ProductName}");
WaveOutEvent soundCard = new WaveOutEvent();
soundCard.DeviceNumber = i;
soundCards.Add(soundCard);
SpeechSynthesizer speechSynthesizer = new SpeechSynthesizer();
speechSynthesizers.Add(speechSynthesizer);
}
// 播放多个文本语音
for (int i = 0; i < soundCards.Count; i++)
{
WaveOutEvent soundCard = soundCards[i];
SpeechSynthesizer speechSynthesizer = speechSynthesizers[i];
string text = texts[i % texts.Count];
// 配置Text-to-Speech引擎
speechSynthesizer.SelectVoiceByHints(VoiceGender.Female);
speechSynthesizer.Rate = -2;
speechSynthesizer.Volume = 100;
// 合成音频数据
var ms = new System.IO.MemoryStream();
speechSynthesizer.SetOutputToWaveStream(ms);
speechSynthesizer.Speak(text);
ms.Position = 0;
// 写入声卡缓冲区
var waveProvider = new RawSourceWaveStream(ms, new WaveFormat(16000, 16, 1));
soundCard.Init(waveProvider);
soundCard.Play();
}
Console.ReadKey();
// 关闭声卡
foreach (var soundCard in soundCards)
{
soundCard.Stop();
soundCard.Dispose();
}
// 关闭Text-to-Speech引擎
foreach (var speechSynthesizer in speechSynthesizers)
{
speechSynthesizer.Dispose();
}
}
}
}
```
该示例首先打开多个声卡,并创建多个实例。然后,它使用SpeechSynthesizer类将多个文本合成为音频数据,并将它们写入对应的声卡缓冲区中。最后,它通过按任意键来关闭声卡并释放资源。
需要注意的是,不同的声卡可能有不同的设置要求,您需要确保每个声卡的设置正确,并且音频数据格式相同。此外,Text-to-Speech引擎的语音合成质量也会影响最终的音质,您需要根据自己的需求选择合适的引擎。
相关推荐
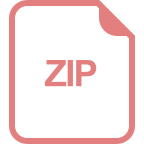
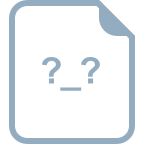
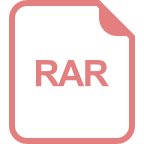
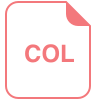
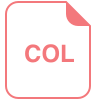
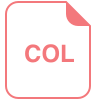
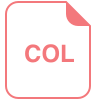
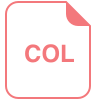








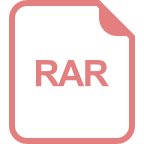