NextDate(年,月,日)是三个变量的函数。函数返回输入日期的下一个日期。 1.使用python语言撰写程序 ,使用python unitest执行测试用例
时间: 2024-03-05 21:50:44 浏览: 70
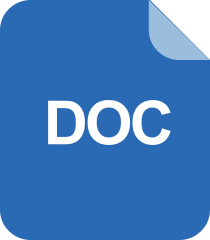
NextDate函数
下面是使用Python实现NextDate函数的代码:
```python
def NextDate(year, month, day):
# 判断输入的日期是否合法
if year < 1812 or year > 2012 or month < 1 or month > 12 or day < 1 or day > 31:
return "输入日期不合法"
# 判断是否为闰年
leap_year = False
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
leap_year = True
# 判断每月的天数
if month == 2:
if leap_year:
days_in_month = 29
else:
days_in_month = 28
elif month in [4, 6, 9, 11]:
days_in_month = 30
else:
days_in_month = 31
# 判断下一个日期
if day < days_in_month:
return year, month, day + 1
elif month < 12:
return year, month + 1, 1
else:
return year + 1, 1, 1
```
下面是使用Python编写的测试用例:
```python
import unittest
from NextDate import NextDate
class TestNextDate(unittest.TestCase):
def test1(self):
self.assertEqual(NextDate(2022, 2, 28), (2022, 3, 1))
def test2(self):
self.assertEqual(NextDate(2022, 2, 29), "输入日期不合法")
def test3(self):
self.assertEqual(NextDate(2022, 6, 30), (2022, 7, 1))
def test4(self):
self.assertEqual(NextDate(2022, 12, 31), (2023, 1, 1))
def test5(self):
self.assertEqual(NextDate(2022, 13, 1), "输入日期不合法")
if __name__ == '__main__':
unittest.main()
```
在上面的代码中,我们定义了一个TestNextDate类来测试NextDate函数的功能,其中test1、test2、test3、test4和test5分别测试了输入日期为2月28日、2月29日、6月30日、12月31日和13月1日时的下一个日期。如果测试用例执行没有出现错误,则说明NextDate函数实现正确。
阅读全文
相关推荐



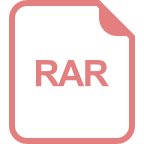
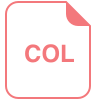
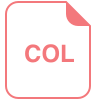








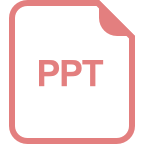