现在你想要对自己心仪的对象告白,利用C语言程序设置了密文,告知对方需要将每个字母往后移动1个才是你要表达的真实原文。现在你要编写程序将你的原文表白例如“LOVE”转换为密文。 ①使用printf与scanf实现 ②使用putchar与getchar实现 c语言
时间: 2024-03-25 14:40:37 浏览: 113
以下是使用 `printf` 与 `scanf` 实现将原文转换为密文的 C 语言程序代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char plaintext[MAX_LEN+1], ciphertext[MAX_LEN+1];
int i, len;
printf("请输入原文:");
scanf("%s", plaintext);
len = strlen(plaintext);
for (i = 0; i < len; i++) {
if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
ciphertext[i] = (plaintext[i] - 'a' + 1) % 26 + 'a';
} else if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
ciphertext[i] = (plaintext[i] - 'A' + 1) % 26 + 'A';
} else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[len] = '\0';
printf("密文为:%s\n", ciphertext);
return 0;
}
```
程序首先通过 `scanf` 获取用户输入的原文字符串,然后遍历该字符串中的每个字符,对于每个字母,将其往后移动1个位置,对于非字母字符则不作处理。具体实现是通过判断字符的 ASCII 编码值来实现的,对于小写字母,其编码值在 'a' 到 'z' 之间,对于大写字母,其编码值在 'A' 到 'Z' 之间。最后,将加密后的字符串输出到屏幕上。
以下是使用 `putchar` 与 `getchar` 实现将原文转换为密文的 C 语言程序代码:
```c
#include <stdio.h>
int main() {
char ch;
printf("请输入原文:");
while ((ch = getchar()) != '\n') {
if (ch >= 'a' && ch <= 'z') {
putchar((ch - 'a' + 1) % 26 + 'a');
} else if (ch >= 'A' && ch <= 'Z') {
putchar((ch - 'A' + 1) % 26 + 'A');
} else {
putchar(ch);
}
}
return 0;
}
```
程序通过 `getchar` 逐个获取用户输入的字符,对于每个字母,将其往后移动1个位置,对于非字母字符则不作处理。具体实现方法同样是通过判断字符的 ASCII 编码值来实现的,对于小写字母,其编码值在 'a' 到 'z' 之间,对于大写字母,其编码值在 'A' 到 'Z' 之间。最后,将加密后的字符逐个输出到屏幕上。
阅读全文
相关推荐
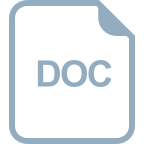
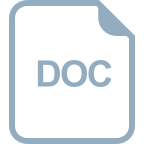
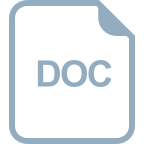
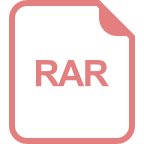














