编写一个程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。
时间: 2024-03-19 07:43:58 浏览: 39
好的,以下是一个Python程序实现您的要求:
```python
import os
import pickle
from Crypto.Cipher import AES
import getpass
# 生成随机密钥
def generate_key():
return os.urandom(16)
# 加密函数
def encrypt(key, plaintext):
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext.encode())
return ciphertext, cipher.nonce, tag
# 解密函数
def decrypt(key, nonce, ciphertext, tag):
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
plaintext = cipher.decrypt(ciphertext)
try:
cipher.verify(tag)
return plaintext.decode()
except:
return None
# 保存密钥和变形码到文件
def save_key(filename, key, nonce):
with open(filename, 'wb') as f:
data = {'key': key, 'nonce': nonce}
pickle.dump(data, f)
# 从文件中读取密钥和变形码
def load_key(filename):
with open(filename, 'rb') as f:
data = pickle.load(f)
key = data['key']
nonce = data['nonce']
return key, nonce
# 加密文字并保存到文件
def save_text(filename, key, plaintext):
ciphertext, nonce, tag = encrypt(key, plaintext)
with open(filename, 'ab') as f:
f.write(ciphertext)
f.write(nonce)
f.write(tag)
# 从文件中读取密文并解密
def load_text(filename, key):
with open(filename, 'rb') as f:
ciphertext = f.read()
nonce = ciphertext[-16:]
tag = ciphertext[-32:-16]
ciphertext = ciphertext[:-32]
plaintext = decrypt(key, nonce, ciphertext, tag)
return plaintext
# 密码验证函数
def verify_password():
password = '123456' # 正确的密码
max_tries = 3 # 最大尝试次数
for i in range(max_tries):
prompt = '请输入密码:' if i == 0 else '密码错误,请重新输入:'
entered_password = getpass.getpass(prompt)
if entered_password == password:
return True
return False
# 主程序
filename = '姓名.dat'
if os.path.exists(filename):
# 文件已经存在,需要验证密码
if verify_password():
# 密码验证通过,读取密钥和变形码
key, nonce = load_key(filename)
# 读取密文并解密
plaintext = load_text(filename, key)
print('文件内容为:', plaintext)
else:
# 密码验证失败,退出程序
print('密码验证失败,程序退出!')
else:
# 文件不存在,需要创建新文件并输入内容
key = generate_key()
nonce = os.urandom(16)
save_key(filename, key, nonce)
plaintext = input('请输入要保存的内容:')
save_text(filename, key, plaintext)
print('文件保存成功!')
```
在运行程序时,如果文件不存在,则会要求输入要保存的内容,并保存到文件中。如果文件已经存在,则会要求输入密码进行验证,验证通过后会读取文件内容并输出。如果密码验证失败,则程序会退出。
需要注意的是,以上程序只是一个简单的示例,实际使用中需要加入更多的安全措施,比如使用哈希函数来保存密码、使用更加安全的加密算法等。
阅读全文
相关推荐



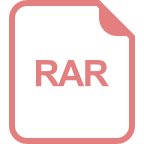
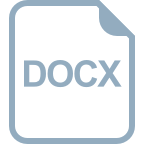
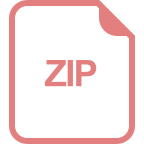
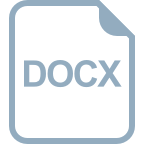
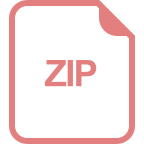
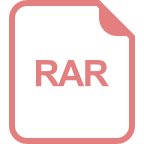
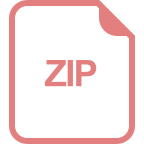
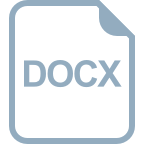