Java使用简单工厂模式设计一个可以创建不同几何形状(如圆形,方形和三角形等)的绘图工具,每个几何元素都有绘制draw(),和擦除erase()两个函数,要求再绘制不支持的几何图形时提示一个UnSupportedShapeException.
时间: 2023-05-17 15:07:30 浏览: 176
好的,这是一个典型的简单工厂模式的应用。首先,我们需要定义一个抽象的几何形状类,包含绘制和擦除两个方法。然后,我们定义具体的几何形状类,如圆形、方形和三角形等,它们都继承自抽象几何形状类,并实现绘制和擦除方法。最后,我们定义一个工厂类,根据传入的参数创建具体的几何形状对象。
下面是Java代码实现:
```java
// 抽象几何形状类
abstract class Shape {
public abstract void draw();
public abstract void erase();
}
// 圆形类
class Circle extends Shape {
public void draw() {
System.out.println("Draw a circle.");
}
public void erase() {
System.out.println("Erase a circle.");
}
}
// 方形类
class Square extends Shape {
public void draw() {
System.out.println("Draw a square.");
}
public void erase() {
System.out.println("Erase a square.");
}
}
// 三角形类
class Triangle extends Shape {
public void draw() {
System.out.println("Draw a triangle.");
}
public void erase() {
System.out.println("Erase a triangle.");
}
}
// 不支持的几何图形异常类
class UnSupportedShapeException extends Exception {
public UnSupportedShapeException(String message) {
super(message);
}
}
// 工厂类
class ShapeFactory {
public static Shape createShape(String shapeType) throws UnSupportedShapeException {
if (shapeType.equalsIgnoreCase("circle")) {
return new Circle();
} else if (shapeType.equalsIgnoreCase("square")) {
return new Square();
} else if (shapeType.equalsIgnoreCase("triangle")) {
return new Triangle();
} else {
throw new UnSupportedShapeException("Unsupported shape type: " + shapeType);
}
}
}
// 测试类
public class Test {
public static void main(String[] args) {
try {
Shape circle = ShapeFactory.createShape("circle");
circle.draw();
circle.erase();
Shape square = ShapeFactory.createShape("square");
square.draw();
square.erase();
Shape triangle = ShapeFactory.createShape("triangle");
triangle.draw();
triangle.erase();
Shape unsupported = ShapeFactory.createShape("rectangle");
unsupported.draw();
unsupported.erase();
} catch (UnSupportedShapeException e) {
System.out.println(e.getMessage());
}
}
}
```
输出结果为:
```
Draw a circle.
Erase a circle.
Draw a square.
Erase a square.
Draw a triangle.
Erase a triangle.
Unsupported shape type: rectangle
```
当我们创建一个不支持的几何图形时,会抛出一个UnSupportedShapeException异常。
阅读全文
相关推荐
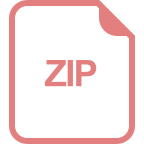

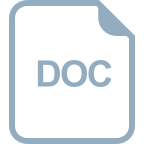
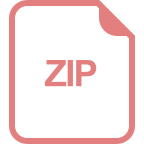
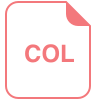
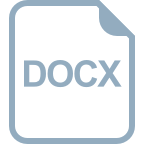
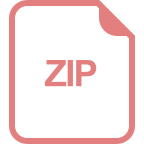
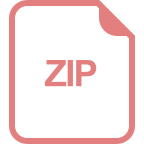
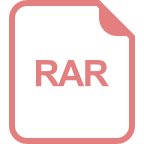
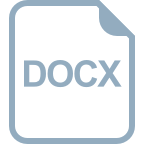
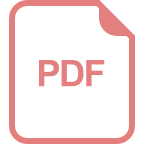
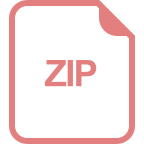
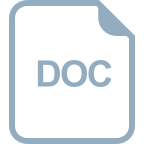
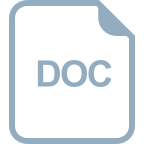
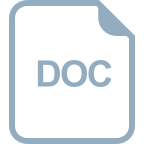
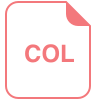
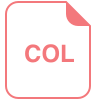