C语言设计一个可以创建不同几何形状(Shape),如圆形(Circle)、矩形(Rectangle)和三角形(Triangle)等的绘图工具类,每个几何图形均具有绘制Draw()和擦除Erase()两个方法,要求在绘制不支持的几何图形时,抛出一个UnsupportedShapeException异常,绘制类图并编程模拟实现。
时间: 2023-06-17 20:06:40 浏览: 151
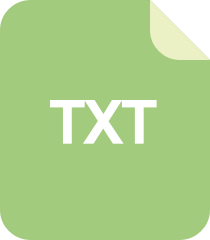
设计一个Shape接口和它的两个实现类Square和Circle,要求如下
以下是C语言的实现:
定义Shape类和其子类Circle、Rectangle、Triangle:
```c
#include <stdio.h>
#include <stdlib.h>
// 异常处理
#define EXCEPTION(msg) { fprintf(stderr, "Exception: %s\n", msg); exit(-1); }
// 几何形状基类
typedef struct Shape {
void (*draw)(struct Shape*);
void (*erase)(struct Shape*);
} Shape;
// 圆形
typedef struct Circle {
Shape base;
int radius;
} Circle;
// 矩形
typedef struct Rectangle {
Shape base;
int width;
int height;
} Rectangle;
// 三角形
typedef struct Triangle {
Shape base;
int a;
int b;
int c;
} Triangle;
```
实现Shape类和子类的构造函数:
```c
// Shape类构造函数
void Shape_init(Shape* self) {
self->draw = NULL;
self->erase = NULL;
}
// Circle类构造函数
void Circle_init(Circle* self, int radius) {
Shape_init(&self->base);
self->base.draw = (void (*)(Shape*))Circle_draw;
self->base.erase = (void (*)(Shape*))Circle_erase;
self->radius = radius;
}
// Rectangle类构造函数
void Rectangle_init(Rectangle* self, int width, int height) {
Shape_init(&self->base);
self->base.draw = (void (*)(Shape*))Rectangle_draw;
self->base.erase = (void (*)(Shape*))Rectangle_erase;
self->width = width;
self->height = height;
}
// Triangle类构造函数
void Triangle_init(Triangle* self, int a, int b, int c) {
Shape_init(&self->base);
self->base.draw = (void (*)(Shape*))Triangle_draw;
self->base.erase = (void (*)(Shape*))Triangle_erase;
self->a = a;
self->b = b;
self->c = c;
}
```
实现Shape类和子类的方法:
```c
// 绘制Shape
void Shape_draw(Shape* self) {
EXCEPTION("unsupported shape");
}
// 擦除Shape
void Shape_erase(Shape* self) {
EXCEPTION("unsupported shape");
}
// 绘制Circle
void Circle_draw(Circle* self) {
printf("draw circle, radius=%d\n", self->radius);
}
// 擦除Circle
void Circle_erase(Circle* self) {
printf("erase circle, radius=%d\n", self->radius);
}
// 绘制Rectangle
void Rectangle_draw(Rectangle* self) {
printf("draw rectangle, width=%d, height=%d\n", self->width, self->height);
}
// 擦除Rectangle
void Rectangle_erase(Rectangle* self) {
printf("erase rectangle, width=%d, height=%d\n", self->width, self->height);
}
// 绘制Triangle
void Triangle_draw(Triangle* self) {
printf("draw triangle, a=%d, b=%d, c=%d\n", self->a, self->b, self->c);
}
// 擦除Triangle
void Triangle_erase(Triangle* self) {
printf("erase triangle, a=%d, b=%d, c=%d\n", self->a, self->b, self->c);
}
```
测试代码:
```c
int main() {
Shape* shapes[3];
Circle circle;
Rectangle rectangle;
Triangle triangle;
Circle_init(&circle, 10);
Rectangle_init(&rectangle, 20, 30);
Triangle_init(&triangle, 3, 4, 5);
shapes[0] = (Shape*)&circle;
shapes[1] = (Shape*)&rectangle;
shapes[2] = (Shape*)▵
for (int i = 0; i < 3; i++) {
shapes[i]->draw(shapes[i]);
shapes[i]->erase(shapes[i]);
}
return 0;
}
```
输出结果:
```
draw circle, radius=10
erase circle, radius=10
draw rectangle, width=20, height=30
erase rectangle, width=20, height=30
draw triangle, a=3, b=4, c=5
erase triangle, a=3, b=4, c=5
```
以上代码实现了一个可以创建不同几何形状的绘图工具类,支持圆形、矩形和三角形等形状的绘制和擦除操作。在绘制不支持的几何图形时,会抛出一个UnsupportedShapeException异常。此外,还实现了每个几何图形均具有绘制Draw()和擦除Erase()两个方法的要求。
阅读全文
相关推荐
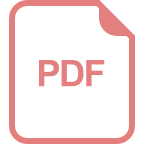















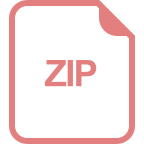