使用工厂模式设计一个可以创建不同几何形状(Shape)
时间: 2024-06-12 07:10:41 浏览: 97
好的,可以使用工厂模式来实现这个需求。首先需要定义一个 Shape 接口,然后定义不同的实现类,如 Circle、Rectangle 等。接着创建一个工厂类 ShapeFactory,其中包含一个静态方法 getShape(String shapeType),根据传入的参数来返回相应的 Shape 实例。
以下是示例代码:
```java
// Shape 接口
public interface Shape {
void draw();
}
// Circle 类
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle.");
}
}
// Rectangle 类
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle.");
}
}
// ShapeFactory 工厂类
public class ShapeFactory {
public static Shape getShape(String shapeType) {
if (shapeType == null) {
return null;
}
if (shapeType.equalsIgnoreCase("CIRCLE")) {
return new Circle();
} else if (shapeType.equalsIgnoreCase("RECTANGLE")) {
return new Rectangle();
}
return null;
}
}
```
使用示例:
```java
Shape circle = ShapeFactory.getShape("CIRCLE");
circle.draw(); // 输出 "Drawing a circle."
Shape rectangle = ShapeFactory.getShape("RECTANGLE");
rectangle.draw(); // 输出 "Drawing a rectangle."
```
阅读全文
相关推荐
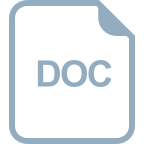
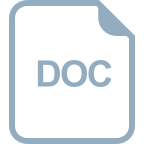
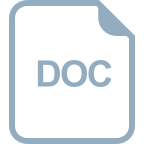








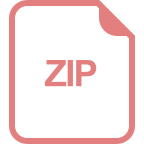
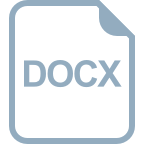
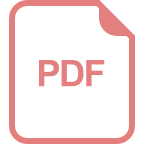
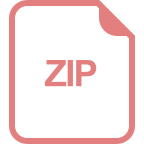
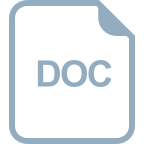
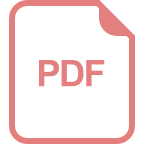