使用简单工厂模式设计一个可以创建不同几何形状(如圆形、矩形和三角形)的绘图工厂,每个几何图形都有计算面积area()和计算周长gride()两个方法,要求在创建不支持的几何图形时,提示一个UnSupportedShapeException。
时间: 2024-11-22 20:48:13 浏览: 19
在使用简单工厂模式设计这样一个几何形状绘图工厂时,首先我们需要创建一个抽象的几何形状接口,例如`IGeometry`:
```java
public interface IGeometry {
double getArea();
double getPerimeter();
}
```
然后分别创建圆形、矩形和三角形的具体实现类,比如`Circle`, `Rectangle`, 和 `Triangle`,它们都实现`IGeometry`接口:
```java
public class Circle implements IGeometry {
private final double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public double getPerimeter() {
return 2 * Math.PI * radius;
}
}
public class Rectangle implements IGeometry {
private final double width;
private final double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
@Override
public double getPerimeter() {
return 2 * (width + height);
}
}
public class Triangle implements IGeometry {
private double base;
private double height;
// 构造函数...
@Override
public double getArea() {
// 计算三角形面积...
}
@Override
public double getPerimeter() {
// 计算三角形周长...
}
}
```
接着,我们创建一个`DrawingFactory`类,它包含一个创建几何形状的对象列表,并通过工厂方法返回对应类型的实例:
```java
public class DrawingFactory {
private static Map<String, Class<? extends IGeometry>> shapesMap = new HashMap<>();
static {
shapesMap.put("circle", Circle.class);
shapesMap.put("rectangle", Rectangle.class);
shapesMap.put("triangle", Triangle.class);
}
public static IGeometry createShape(String shapeType, Object... args) throws UnSupportedShapeException {
if (!shapesMap.containsKey(shapeType)) {
throw new UnSupportedShapeException("Unsupported shape type: " + shapeType);
}
try {
Constructor<?> constructor = shapesMap.get(shapeType).getConstructor(args.getClass());
return (IGeometry) constructor.newInstance(args);
} catch (NoSuchMethodException | InstantiationException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException(e);
}
}
}
```
现在你可以像这样使用这个工厂:
```java
try {
IGeometry circle = DrawingFactory.createShape("circle", 5);
System.out.println(circle.getArea()); // 输出圆的面积
IGeometry rectangle = DrawingFactory.createShape("rectangle", 4, 6);
System.out.println(rectangle.getArea()); // 输出矩形的面积
IGeometry triangle = DrawingFactory.createShape("triangle", 3, 4); // 提示 UnSupportedShapeException
} catch (UnSupportedShapeException e) {
e.printStackTrace();
}
```
阅读全文
相关推荐
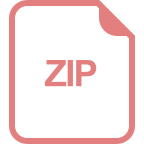
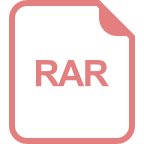





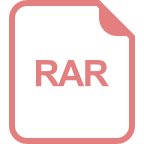
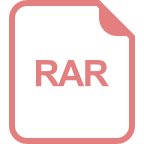
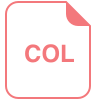
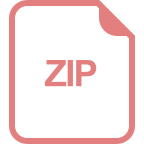
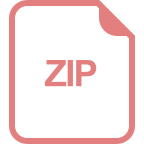
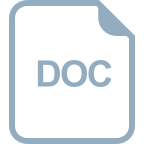
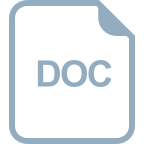
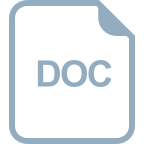
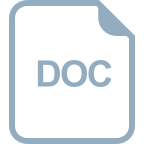
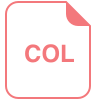
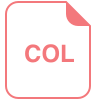
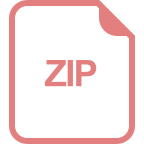