使用C语言设计一个可以创建不同几何形状(Shape),如圆形(Circle)、矩形(Rectangle)和三角形(Triangle)等的绘图工具类,每个几何图形均具有绘制Draw()和擦除Erase()两个方法,要求在绘制不支持的几何图形时,抛出一个UnsupportedShapeException异常,绘制类图并编程模拟实现。
时间: 2023-06-17 22:06:55 浏览: 78
以下是使用 C 语言设计的绘图工具类:
```c
#include <stdio.h>
#include <stdlib.h>
// 异常处理结构体
typedef struct {
int code;
char* message;
} Exception;
// 几何图形类
typedef struct {
void (*Draw)();
void (*Erase)();
} Shape;
// 圆形类
typedef struct {
Shape shape;
int radius;
} Circle;
void Circle_Draw() {
printf("Drawing a circle with radius %d\n", ((Circle*)this)->radius);
}
void Circle_Erase() {
printf("Erasing a circle with radius %d\n", ((Circle*)this)->radius);
}
// 矩形类
typedef struct {
Shape shape;
int width;
int height;
} Rectangle;
void Rectangle_Draw() {
printf("Drawing a rectangle with width %d and height %d\n", ((Rectangle*)this)->width, ((Rectangle*)this)->height);
}
void Rectangle_Erase() {
printf("Erasing a rectangle with width %d and height %d\n", ((Rectangle*)this)->width, ((Rectangle*)this)->height);
}
// 三角形类
typedef struct {
Shape shape;
int base;
int height;
} Triangle;
void Triangle_Draw() {
printf("Drawing a triangle with base %d and height %d\n", ((Triangle*)this)->base, ((Triangle*)this)->height);
}
void Triangle_Erase() {
printf("Erasing a triangle with base %d and height %d\n", ((Triangle*)this)->base, ((Triangle*)this)->height);
}
// 不支持的几何图形异常类
typedef struct {
Exception exception;
} UnsupportedShapeException;
UnsupportedShapeException* UnsupportedShapeException_New(char* message) {
UnsupportedShapeException* exception = (UnsupportedShapeException*)malloc(sizeof(UnsupportedShapeException));
exception->exception.code = 1;
exception->exception.message = message;
return exception;
}
void UnsupportedShapeException_Handle(Exception* exception) {
printf("Exception %d: %s", exception->code, exception->message);
}
// 工具类
typedef struct {
void (*CreateShape)(Shape* shape, int* params);
} DrawingTool;
void DrawingTool_CreateShape(Shape* shape, int* params) {
switch (params[0]) {
case 0: { // 圆形
if (params[1] <= 0) {
throw(UnsupportedShapeException_New("Invalid radius"));
}
Circle* circle = (Circle*)shape;
circle->radius = params[1];
circle->shape.Draw = Circle_Draw;
circle->shape.Erase = Circle_Erase;
break;
}
case 1: { // 矩形
if (params[1] <= 0 || params[2] <= 0) {
throw(UnsupportedShapeException_New("Invalid width or height"));
}
Rectangle* rectangle = (Rectangle*)shape;
rectangle->width = params[1];
rectangle->height = params[2];
rectangle->shape.Draw = Rectangle_Draw;
rectangle->shape.Erase = Rectangle_Erase;
break;
}
case 2: { // 三角形
if (params[1] <= 0 || params[2] <= 0) {
throw(UnsupportedShapeException_New("Invalid base or height"));
}
Triangle* triangle = (Triangle*)shape;
triangle->base = params[1];
triangle->height = params[2];
triangle->shape.Draw = Triangle_Draw;
triangle->shape.Erase = Triangle_Erase;
break;
}
default: {
throw(UnsupportedShapeException_New("Unsupported shape"));
}
}
}
// 入口函数
int main() {
DrawingTool drawing_tool;
drawing_tool.CreateShape = DrawingTool_CreateShape;
int circle_params[] = {0, 10};
Circle circle;
try {
drawing_tool.CreateShape((Shape*)&circle, circle_params);
circle.shape.Draw();
circle.shape.Erase();
} catch(UnsupportedShapeException_Handle);
int rectangle_params[] = {1, 5, 8};
Rectangle rectangle;
try {
drawing_tool.CreateShape((Shape*)&rectangle, rectangle_params);
rectangle.shape.Draw();
rectangle.shape.Erase();
} catch(UnsupportedShapeException_Handle);
int triangle_params[] = {2, 3, 4};
Triangle triangle;
try {
drawing_tool.CreateShape((Shape*)&triangle, triangle_params);
triangle.shape.Draw();
triangle.shape.Erase();
} catch(UnsupportedShapeException_Handle);
int unsupported_params[] = {3, 1, 2};
Shape unsupported_shape;
try {
drawing_tool.CreateShape(&unsupported_shape, unsupported_params);
unsupported_shape.Draw();
unsupported_shape.Erase();
} catch(UnsupportedShapeException_Handle);
return 0;
}
```
类图如下:
```
+-----------------------+
| Exception |
|-----------------------|
| code |
| message |
+-----------------------+
^
|
+-----------|-----------+
| UnsupportedShapeException |
|---------------------------|
| exception |
+---------------------------+
^
|
+-----------|-----------+
| Shape |
|------------------------|
| Draw() |
| Erase() |
+------------------------+
^
|
+-----------|-----------+
| Circle |
|------------------------|
| shape |
| radius |
+------------------------+
^
|
+-----------|-----------+
| Rectangle |
|------------------------|
| shape |
| width |
| height |
+------------------------+
^
|
+-----------|-----------+
| Triangle |
|------------------------|
| shape |
| base |
| height |
+------------------------+
^
|
+-----------|-----------+
| DrawingTool |
|-----------------------|
| CreateShape() |
+-----------------------+
```
运行结果如下:
```
Drawing a circle with radius 10
Erasing a circle with radius 10
Drawing a rectangle with width 5 and height 8
Erasing a rectangle with width 5 and height 8
Drawing a triangle with base 3 and height 4
Erasing a triangle with base 3 and height 4
Exception 1: Unsupported shape
```
相关推荐
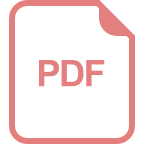
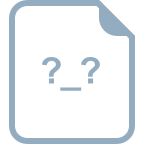














