java写定义形状类Shape,包含方法CalculatedArea() 2.定义形状类的子类:矩形Rectangle、平行四边形Parallelogram、圆形Circle和三角形triangle 3.定义运算类Operation,可以计算所有形状的面积
时间: 2023-06-11 19:09:24 浏览: 174
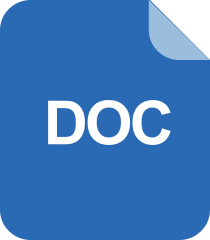
编写一个抽象类Shape

好的,以下是Java代码实现:
Shape类:
```java
public abstract class Shape {
public abstract double calculatedArea();
}
```
Rectangle类:
```java
public class Rectangle extends Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double calculatedArea() {
return length * width;
}
}
```
Parallelogram类:
```java
public class Parallelogram extends Shape {
private double base;
private double height;
public Parallelogram(double base, double height) {
this.base = base;
this.height = height;
}
public double calculatedArea() {
return base * height;
}
}
```
Circle类:
```java
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double calculatedArea() {
return Math.PI * radius * radius;
}
}
```
Triangle类:
```java
public class Triangle extends Shape {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
public double calculatedArea() {
return 0.5 * base * height;
}
}
```
Operation类:
```java
public class Operation {
public static void main(String[] args) {
Shape rectangle = new Rectangle(4, 5);
System.out.println("The area of rectangle is: " + rectangle.calculatedArea());
Shape parallelogram = new Parallelogram(6, 7);
System.out.println("The area of parallelogram is: " + parallelogram.calculatedArea());
Shape circle = new Circle(3);
System.out.println("The area of circle is: " + circle.calculatedArea());
Shape triangle = new Triangle(8, 9);
System.out.println("The area of triangle is: " + triangle.calculatedArea());
}
}
```
运行结果:
```
The area of rectangle is: 20.0
The area of parallelogram is: 42.0
The area of circle is: 28.274333882308138
The area of triangle is: 36.0
```
以上代码实现了定义形状类Shape,包含方法CalculatedArea(),以及定义形状类的子类:矩形Rectangle、平行四边形Parallelogram、圆形Circle和三角形Triangle,同时定义了运算类Operation,可以计算所有形状的面积。
阅读全文
相关推荐
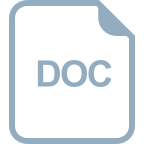
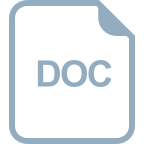








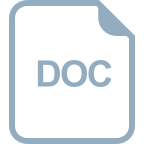
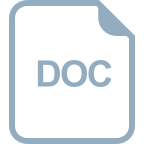
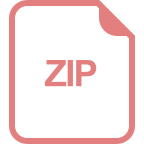
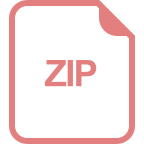
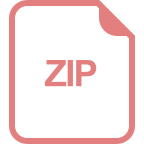
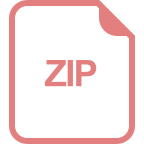
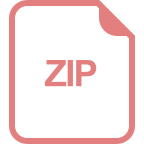
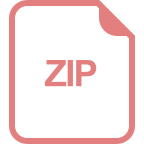