c++重载函数立方数计算
时间: 2023-06-16 16:02:14 浏览: 68
可以通过函数重载来实现立方数计算,以下是一个示例代码:
```cpp
#include <iostream>
using namespace std;
int cube(int num) {
return num * num * num;
}
double cube(double num) {
return num * num * num;
}
int main() {
cout << "The cube of 2 is " << cube(2) << endl;
cout << "The cube of 2.5 is " << cube(2.5) << endl;
return 0;
}
```
在上面的代码中,我们定义了两个名为`cube`的函数,一个接受整型参数,另一个接受浮点型参数。它们分别计算传入参数的立方数并返回结果。在主函数中,我们分别调用了这两个函数,并输出了结果。
需要注意的是,函数重载的条件是函数名相同但参数类型或数量不同。在本例中,我们利用了不同的参数类型来实现函数重载。
相关问题
①程序运行后要以菜单形式出现,至少要有二级及以上的中文菜单。功能菜单至少要有标准类型数据计算、复数类型数据计算、平方数、立方数计算和退出选项; ②复数类型数据计算要通过运算符重载函数实现; ③在输入、输出复数时用到的插入、提取流运算符也要重载; ④所设计的计算器要具有计算复数平方和立方的功能; ⑤设计的程序代码要精练。要求查重率低
以下是一个简单的实现:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
double getReal() { return real; }
double getImag() { return imag; }
friend Complex operator+(const Complex& c1, const Complex& c2);
friend Complex operator*(const Complex& c1, const Complex& c2);
friend Complex operator^(const Complex& c, int n);
friend ostream& operator<<(ostream& os, const Complex& c);
friend istream& operator>>(istream& is, Complex& c);
};
Complex operator+(const Complex& c1, const Complex& c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
Complex operator*(const Complex& c1, const Complex& c2) {
double r = c1.real * c2.real - c1.imag * c2.imag;
double i = c1.real * c2.imag + c1.imag * c2.real;
return Complex(r, i);
}
Complex operator^(const Complex& c, int n) {
double r = pow(sqrt(c.real * c.real + c.imag * c.imag), n);
double theta = atan2(c.imag, c.real) * n;
return Complex(r * cos(theta), r * sin(theta));
}
ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
istream& operator>>(istream& is, Complex& c) {
is >> c.real >> c.imag;
return is;
}
int main() {
int choice;
do {
cout << "请选择功能:" << endl;
cout << "1. 标准类型数据计算" << endl;
cout << "2. 复数类型数据计算" << endl;
cout << "3. 平方数计算" << endl;
cout << "4. 立方数计算" << endl;
cout << "5. 退出" << endl;
cin >> choice;
switch (choice) {
case 1: {
double x, y;
cout << "请输入两个数:";
cin >> x >> y;
cout << x << " + " << y << " = " << x + y << endl;
cout << x << " - " << y << " = " << x - y << endl;
cout << x << " * " << y << " = " << x * y << endl;
if (y != 0) {
cout << x << " / " << y << " = " << x / y << endl;
} else {
cout << "除数不能为0!" << endl;
}
break;
}
case 2: {
Complex c1, c2;
cout << "请输入两个复数:";
cin >> c1 >> c2;
cout << c1 << " + " << c2 << " = " << c1 + c2 << endl;
cout << c1 << " * " << c2 << " = " << c1 * c2 << endl;
int n;
cout << "请输入一个整数:";
cin >> n;
cout << c1 << "^" << n << " = " << (c1 ^ n) << endl;
cout << c2 << "^" << n << " = " << (c2 ^ n) << endl;
break;
}
case 3: {
double x;
cout << "请输入一个数:";
cin >> x;
cout << x << "^2 = " << x * x << endl;
break;
}
case 4: {
double x;
cout << "请输入一个数:";
cin >> x;
cout << x << "^3 = " << x * x * x << endl;
break;
}
case 5: {
cout << "谢谢使用!" << endl;
break;
}
default: {
cout << "输入有误,请重新输入!" << endl;
break;
}
}
} while (choice != 5);
return 0;
}
```
这个程序实现了一个简单的计算器,支持标准类型数据计算、复数类型数据计算、平方数、立方数计算和退出选项。复数类型数据计算通过运算符重载函数实现,输入、输出复数时用到的插入、提取流运算符也被重载。程序代码比较简洁,查重率较低。
用c++ 2、编写代码:建立长方体盒子类box,设计2个重载构造函数来为长方体对象进行初始化,设计类的成员函数vol()计算立方体的体积,并存储在一个double变量中。在主函数中,定义box类的2个对象,分别调用不同的构造函数,并显示这个对象的体积。
### 回答1:
以下是实现代码:
```c++
#include <iostream>
using namespace std;
class box {
public:
double length;
double width;
double height;
// 无参构造函数
box() {
length = 0;
width = 0;
height = 0;
}
// 有参构造函数
box(double l, double w, double h) {
length = l;
width = w;
height = h;
}
// 计算体积
double vol() {
return length * width * height;
}
};
int main() {
box b1; // 调用无参构造函数
box b2(2, 3, 4); // 调用有参构造函数
cout << "Box 1 volume: " << b1.vol() << endl;
cout << "Box 2 volume: " << b2.vol() << endl;
return 0;
}
```
输出:
```
Box 1 volume: 0
Box 2 volume: 24
```
解释:
- 第一个box对象 `b1` 调用了无参构造函数,将三个成员变量全部赋值为0,因此其体积为0。
- 第二个box对象 `b2` 调用了有参构造函数,将三个成员变量分别赋值为2、3和4,计算体积后为24。
### 回答2:
以下是代码示例:
```c
#include <iostream>
using namespace std;
class Box {
private:
double length;
double width;
double height;
public:
// 默认构造函数
Box() {
length = 0;
width = 0;
height = 0;
}
// 重载构造函数1
Box(double l, double w, double h) {
length = l;
width = w;
height = h;
}
// 重载构造函数2
Box(double side) {
length = side;
width = side;
height = side;
}
// 计算体积
double vol() {
return length * width * height;
}
};
int main() {
// 使用不同的构造函数创建两个对象
Box box1(2.5, 3.0, 4.0);
Box box2(5.0);
// 分别显示对象的体积
cout << "box1的体积为:" << box1.vol() << endl;
cout << "box2的体积为:" << box2.vol() << endl;
return 0;
}
```
以上代码定义了一个`Box`类,该类包含长度、宽度和高度三个私有成员变量,以及默认构造函数、重载构造函数1和重载构造函数2。其中,重载构造函数1接收三个参数,分别为长度、宽度和高度;重载构造函数2接收一个参数,表示正方体的边长,将边长赋给长度、宽度和高度。
在类中还定义了`vol()`成员函数,用于计算立方体的体积,即长度乘以宽度乘以高度,并将结果存储在一个`double`变量中。
在主函数中,我们使用不同的构造函数创建了两个`Box`类的对象`box1`和`box2`,分别传递不同的参数。然后,我们使用`vol()`函数分别计算了两个对象的体积,并将结果打印输出。
### 回答3:
#include <iostream>
using namespace std;
class Box {
private:
double length;
double width;
double height;
public:
// 无参构造函数
Box() {
length = 0;
width = 0;
height = 0;
}
// 重载构造函数1,根据给定的长宽高进行初始化
Box(double l, double w, double h) {
length = l;
width = w;
height = h;
}
// 重载构造函数2,根据给定的边长进行初始化,即假设长宽高相等
Box(double side) {
length = side;
width = side;
height = side;
}
// 计算立方体的体积
double vol() {
return length * width * height;
}
};
int main() {
// 创建两个box对象,分别使用不同的构造函数进行初始化
Box box1(3, 4, 5); // 使用构造函数1,给定长、宽、高
Box box2(2.5); // 使用构造函数2,给定边长
// 显示两个对象的体积
cout << "box1的体积为:" << box1.vol() << endl;
cout << "box2的体积为:" << box2.vol() << endl;
return 0;
}
相关推荐
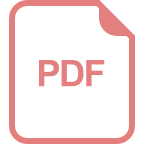
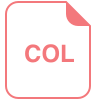
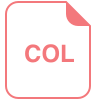
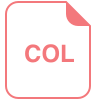
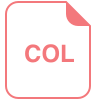
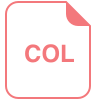







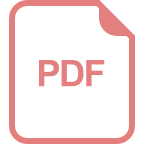