windows下x64与x86共享内存
时间: 2024-01-21 12:04:45 浏览: 27
在Windows下,x64和x86共享内存的方式是一样的。以下是一个简单的示例代码:
```
#include <windows.h>
int main()
{
HANDLE hMapFile;
LPCTSTR pBuf;
hMapFile = CreateFileMapping(
INVALID_HANDLE_VALUE, // Use paging file
NULL, // Default security
PAGE_READWRITE, // Read/write access
0, // Maximum object size (high-order DWORD)
1024, // Maximum object size (low-order DWORD)
L"Local\\MyFileMappingObject"); // Name of the mapping object
pBuf = (LPTSTR)MapViewOfFile(
hMapFile, // Handle to mapping object
FILE_MAP_ALL_ACCESS, // Read/write permission
0,
0,
1024);
CopyMemory((PVOID)pBuf, "Hello, shared memory!", sizeof("Hello, shared memory!"));
// Unmap the shared memory
UnmapViewOfFile(pBuf);
// Close the handle to the mapping object
CloseHandle(hMapFile);
return 0;
}
```
这段代码创建了一个大小为1024字节的共享内存对象,并将其命名为"Local\MyFileMappingObject"。然后它映射到进程的地址空间中,并使用CopyMemory函数将字符串"Hello, shared memory!"写入共享内存。最后,它取消映射共享内存对象并关闭句柄。
其他进程可以通过使用相同的名称打开共享内存对象来访问该共享内存。例如,以下代码打开相同的共享内存并读取其中的数据:
```
#include <windows.h>
int main()
{
HANDLE hMapFile;
LPCTSTR pBuf;
hMapFile = OpenFileMapping(
FILE_MAP_ALL_ACCESS, // Read/write permission
FALSE, // Do not inherit the name
L"Local\\MyFileMappingObject"); // Name of the mapping object
pBuf = (LPTSTR)MapViewOfFile(
hMapFile, // Handle to mapping object
FILE_MAP_ALL_ACCESS, // Read/write permission
0,
0,
1024);
printf("Data read from shared memory: %s\n", pBuf);
// Unmap the shared memory
UnmapViewOfFile(pBuf);
// Close the handle to the mapping object
CloseHandle(hMapFile);
return 0;
}
```
这段代码打开了相同的共享内存对象,并通过MapViewOfFile函数映射到进程的地址空间中。然后,它读取共享内存中的数据并将其打印到控制台上。最后,它取消映射共享内存对象并关闭句柄。
需要注意的是,共享内存对象的名称必须以"Local\"开头,否则将无法在多个进程之间共享。此外,不同的进程之间应该协调好共享内存的读写顺序,以避免数据竞争和死锁等问题。
相关推荐
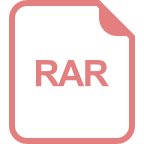
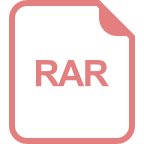
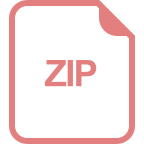














