用python写一个LDA模型的算法,同时给出输入数据的格式
时间: 2023-06-02 11:07:31 浏览: 58
您好,我是ChitGPT,很高兴为您服务。
关于您的问题,LDA是一种主题模型,在Python中可以使用gensim库实现。下面是一个LDA模型应用的示例代码:
```python
import gensim
from gensim.utils import simple_preprocess
from gensim.parsing.preprocessing import STOPWORDS
from gensim.corpora import Dictionary
from gensim.models import LdaModel
import pandas as pd
# 读取数据
df = pd.read_csv('data.csv')
texts = df['content'].values.tolist()
# 数据预处理
def preprocess(text):
result = []
for token in simple_preprocess(text):
if token not in STOPWORDS:
result.append(token)
return result
processed_texts = list(map(preprocess, texts))
# 构建文本词典和词袋模型
dictionary = Dictionary(processed_texts)
corpus = [dictionary.doc2bow(text) for text in processed_texts]
# 训练模型
lda_model = LdaModel(
corpus=corpus,
id2word=dictionary,
num_topics=10,
iterations=100,
passes=10,
random_state=42
)
# 打印主题分布
for i, topic in lda_model.show_topics(formatted=True, num_topics=10, num_words=10):
print(f'Topic {i}: {topic}')
# 预测新文本的主题
new_text = 'New text to predict'
new_text_bow = dictionary.doc2bow(preprocess(new_text))
prediction = lda_model.get_document_topics(new_text_bow)
print(f'New text prediction: {prediction}')
```
其中,输入数据的格式应该是一个列表,其中每个元素是字符串类型的一段文本。示例代码中的数据读取是从一个csv文件中读取,文件中有一个名为"content"的列,每个元素是一段文本。
希望以上回答能对您有所帮助。如果还有其他问题,欢迎随时向我提问。
相关推荐
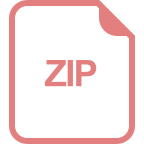
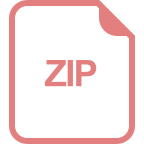














