Traceback (most recent call last): File "D:\PycharmProjects\pythonProject2\main.py", line 44, in <module> output = model_res(images) File "D:\ProgramData\Anaconda3\lib\site-packages\torch\nn\modules\module.py", line 1110, in _call_impl return forward_call(*input, **kwargs) File "D:\ProgramData\Anaconda3\lib\site-packages\timm\models\resnet.py", line 541, in forward x = self.forward_features(x) File "D:\ProgramData\Anaconda3\lib\site-packages\timm\models\resnet.py", line 520, in forward_features x = self.conv1(x) File "D:\ProgramData\Anaconda3\lib\site-packages\torch\nn\modules\module.py", line 1110, in _call_impl return forward_call(*input, **kwargs) File "D:\ProgramData\Anaconda3\lib\site-packages\torch\nn\modules\conv.py", line 447, in forward return self._conv_forward(input, self.weight, self.bias) File "D:\ProgramData\Anaconda3\lib\site-packages\torch\nn\modules\conv.py", line 443, in _conv_forward return F.conv2d(input, weight, bias, self.stride, TypeError: conv2d() received an invalid combination of arguments - got (list, Parameter, NoneType, tuple, tuple, tuple, int), but expected one of: * (Tensor input, Tensor weight, Tensor bias, tuple of ints stride, tuple of ints padding, tuple of ints dilation, int groups) didn't match because some of the arguments have invalid types: (!list!, !Parameter!, !NoneType!, !tuple!, !tuple!, !tuple!, int) * (Tensor input, Tensor weight, Tensor bias, tuple of ints stride, str padding, tuple of ints dilation, int groups) didn't match because some of the arguments have invalid types: (!list!, !Parameter!, !NoneType!, !tuple!, !tuple!, !tuple!, int)
时间: 2023-11-28 12:04:00 浏览: 286
这是一个 Python 的错误追踪(Traceback),它告诉我们在运行 Python 代码时发生了错误。根据 Traceback 的提示,我们可以看到这个错误是在执行模型推理时出现的,具体而言是在执行模型的第一个卷积层时出现的。错误信息显示,conv2d() 函数接收到了一个无效的参数组合,期望的参数类型是 (Tensor input, Tensor weight, Tensor bias, tuple of ints stride, tuple of ints padding, tuple of ints dilation, int groups) 或者 (Tensor input, Tensor weight, Tensor bias, tuple of ints stride, str padding, tuple of ints dilation, int groups),但是实际传入的参数类型是 (list, Parameter, NoneType, tuple, tuple, tuple, int)。根据错误提示,推测可能是输入的数据类型不正确,或者参数传递的方式有误。建议检查代码实现中与输入相关的代码,确保输入数据的类型和形状正确。
相关问题
Traceback (most recent call last): File "D:\PycharmProjects\pythonProject2\main.py", line 47, in <module> models(input) TypeError: 'module' object is not callable
这个错误提示表明你在尝试调用 `models` 模块,但是这个模块本身并不是一个可调用对象。
如果你想要使用 `models` 模块中的某个模型,你需要首先创建一个模型实例,然后对这个模型实例进行调用。以下是一个使用 `resnet18` 模型进行图像分类的示例代码:
```python
import torch
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
# 创建模型实例
model = models.resnet18()
# 加载预训练权重
model.load_state_dict(torch.load("resnet18.pth"))
# 切换为评估模式
model.eval()
# 加载图像
image = Image.open("image.jpg")
# 对图像进行预处理
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(
mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225]
)
])
input = transform(image).unsqueeze(0)
# 使用模型进行预测
output = model(input)
# 输出预测结果
print(output)
```
在这个示例中,我们首先创建了一个 `resnet18` 模型实例,并加载了预训练权重。然后,我们将模型切换为评估模式,并加载了一张图像。接下来,我们对图像进行了预处理,并将其作为输入传给模型进行预测。最后,我们输出了预测结果。
请注意,这里的 `models` 模块并没有被直接调用。我们只是从这个模块中导入了 `resnet18` 模型,并使用它创建了一个模型实例。
Traceback (most recent call last): File "D:\PycharmProjects\pythonProject2\main.py", line 21, in <module> models(input) TypeError: 'module' object is not callable
这个错误提示通常是因为你正在尝试对一个模块对象进行调用,而不是模块中的某个函数、类或方法。在你的代码中,你将一个模块对象'models'作为参数传递给一个函数,而不是调用模块中的某个函数或类。因此,Python解释器无法理解你的意图,抛出了这个异常。
如果你想要使用PyTorch中的预训练模型对图像进行分类,可以使用以下代码:
```python
import torch
import torchvision
from torchvision import transforms
# 定义预处理函数
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
# 加载数据集
dataset = torchvision.datasets.ImageFolder('D:/tupianshuju/erfenlei', transform=preprocess)
# 加载预训练的ResNet18模型
model = torchvision.models.resnet18(pretrained=True)
# 将模型设置为评估模式
model.eval()
# 将输入张量传递给模型进行前向传播
input_tensor = dataset[0][0]
input_tensor = input_tensor.unsqueeze(0)
output = model(input_tensor)
# 输出分类结果
_, predicted = torch.max(output.data, 1)
print('Predicted class index:', predicted.item())
```
在这个示例代码中,我们使用了PyTorch官方提供的ImageFolder类加载图像数据集,并使用预定义的预处理函数对图像进行预处理。然后,我们加载了预训练的ResNet18模型,并将模型设置为评估模式。最后,我们将输入张量传递给模型进行前向传播,并使用torch.max方法找到输出张量中的最大值及其对应的索引,即为预测的类别。
阅读全文
相关推荐
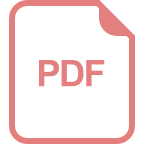
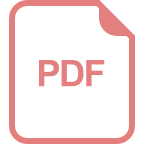
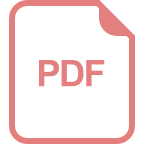














