编写一个函数 day_of_month(year, month) 编写程序输入年(year)、月(month),调用该函数,返回该年份该月的天数,输出返回的天数。 公历闰年的计算方法为: 年份能被4整除且不能被100整除的为闰年 或者,年份能被400整除的是闰年。
时间: 2024-02-12 21:06:09 浏览: 71
好的,我来为你编写这个函数。
```python
def day_of_month(year, month):
# 判断是否为闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
days_of_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31] # 闰年的每月天数
else:
days_of_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31] # 平年的每月天数
return days_of_month[month-1] # 返回该年份该月的天数
```
调用该函数的方式如下:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
days = day_of_month(year, month)
print("{}年{}月的天数为:{}".format(year, month, days))
```
注意,该函数只能计算公历的天数,对于其他历法(如农历、阴历)是无法处理的。
相关问题
【问题描述】 编写一个函数 day_of_month(year, month) 编写程序输入年(year)、月(month),调用该函数,返回该年份该月的天数,输出返回的天数。 公历闰年的计算方法为: 年份能被4整除且不能被100整除的为闰年 或者,年份能被400整除的是闰年。 【输入描述】 共一行。有两个整数,第一个是年份,第二个是月份。年份和月份之间以一个空格隔开。 【输出描述】 输出该年该月的天数。 【输入示例】 2017 11 【输出示例】 30
以下是实现该函数的 Python 代码:
```python
def is_leap_year(year):
"""
判断是否为闰年
"""
return (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0)
def day_of_month(year, month):
"""
计算该年该月的天数
"""
if month in {1, 3, 5, 7, 8, 10, 12}:
return 31
elif month in {4, 6, 9, 11}:
return 30
elif month == 2:
if is_leap_year(year):
return 29
else:
return 28
# 测试代码
year, month = map(int, input().split())
print(day_of_month(year, month))
```
函数 `day_of_month(year, month)` 根据每个月的天数进行判断,需要注意的是二月份的天数需要判断是否为闰年。函数 `is_leap_year(year)` 判断是否为闰年。最后进行输入输出测试即可。
用c语言编程自定义1个函数day_of_year(year, month, day),计算并返回年year、月month和日day对应的是该年的第几天。 函数接口定义: int day_of_year(year, month, day); year, month, day分别为输入的年,月,日 裁判测试程序样例: #include<stdio.h> int day_of_year(int year, int month, int day); int main(void) { int year,month,day; scanf("%d%d%d",&year,&month,&day); printf("%d",day_of_year(year,month,day)); return 0; } /* 请在这里填写答案 */ 输入样例: 2000 3 1 输出样例: 61
以下是实现函数day_of_year的代码:
```
#include <stdio.h>
int day_of_year(int year, int month, int day) {
int days_in_month[] = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
int i, days = 0;
// 判断是否为闰年
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
days_in_month[1] = 29;
}
// 计算天数
for (i = 0; i < month - 1; i++) {
days += days_in_month[i];
}
days += day;
return days;
}
int main(void) {
int year, month, day;
scanf("%d%d%d", &year, &month, &day);
printf("%d", day_of_year(year, month, day));
return 0;
}
```
该函数首先判断给定的年份是否为闰年,根据闰年的情况修改二月的天数。然后根据输入的月份和天数计算出该日期是该年的第几天,最后返回这个天数。在主函数中,读入年、月、日,调用day_of_year函数计算该日期是该年的第几天,并输出结果。
阅读全文
相关推荐
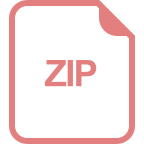











