c语言代码编写两个函数,其功能分别为: ①求N个整数的次大值和次小值。 ②求两个整数的最大公约数和最小公倍数。 输人10个整数,调用函数求它们的次大值和次小值,及次大值和次小值的最大公约数和最小公倍数。 要求:这两个函数和主函数分属3个文件。
时间: 2024-05-06 22:16:42 浏览: 9
以下是代码实现,分别保存为三个文件:
1. main.c
```c
#include <stdio.h>
#include "second_largest_and_smallest.h"
#include "gcd_and_lcm.h"
#define N 10
int main() {
int arr[N];
printf("Please enter %d integers:\n", N);
for (int i = 0; i < N; i++) {
scanf("%d", &arr[i]);
}
int second_largest, second_smallest;
find_second_largest_and_smallest(arr, N, &second_largest, &second_smallest);
printf("Second largest: %d\nSecond smallest: %d\n", second_largest, second_smallest);
int a, b;
printf("Please enter two integers:\n");
scanf("%d%d", &a, &b);
int gcd = find_gcd(a, b);
int lcm = find_lcm(a, b);
printf("GCD: %d\nLCM: %d\n", gcd, lcm);
return 0;
}
```
2. second_largest_and_smallest.h
```c
#ifndef SECOND_LARGEST_AND_SMALLEST_H
#define SECOND_LARGEST_AND_SMALLEST_H
void find_second_largest_and_smallest(int arr[], int n, int *second_largest, int *second_smallest);
#endif //SECOND_LARGEST_AND_SMALLEST_H
```
3. second_largest_and_smallest.c
```c
#include "second_largest_and_smallest.h"
void find_second_largest_and_smallest(int arr[], int n, int *second_largest, int *second_smallest) {
// Initialize the largest and smallest variables to be the first element in the array
int largest = arr[0], smallest = arr[0];
// Initialize the second largest and smallest variables to be the same as largest and smallest
*second_largest = largest;
*second_smallest = smallest;
for (int i = 1; i < n; i++) {
if (arr[i] > largest) {
// If the current element is larger than the largest, update the second largest and largest
*second_largest = largest;
largest = arr[i];
} else if (arr[i] > *second_largest) {
// If the current element is between the largest and second largest, update the second largest
*second_largest = arr[i];
}
if (arr[i] < smallest) {
// If the current element is smaller than the smallest, update the second smallest and smallest
*second_smallest = smallest;
smallest = arr[i];
} else if (arr[i] < *second_smallest) {
// If the current element is between the smallest and second smallest, update the second smallest
*second_smallest = arr[i];
}
}
}
```
4. gcd_and_lcm.h
```c
#ifndef GCD_AND_LCM_H
#define GCD_AND_LCM_H
int find_gcd(int a, int b);
int find_lcm(int a, int b);
#endif //GCD_AND_LCM_H
```
5. gcd_and_lcm.c
```c
#include "gcd_and_lcm.h"
int find_gcd(int a, int b) {
if (b == 0) {
return a;
}
return find_gcd(b, a % b);
}
int find_lcm(int a, int b) {
int gcd = find_gcd(a, b);
return a * b / gcd;
}
```
相关推荐
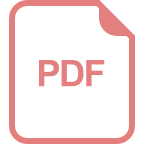
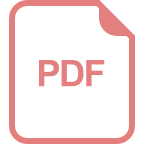













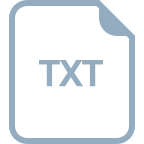
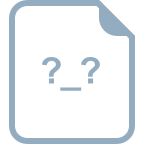